list.steam转map
时间: 2023-10-17 16:33:47 浏览: 56
你可以使用`stream`流中的`collect()`方法将`List`转换为`Map`。下面是一个示例代码:
```java
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
List<String> list = List.of("A", "B", "C");
Map<String, Integer> map = list.stream()
.collect(Collectors.toMap(s -> s, s -> s.length()));
System.out.println(map);
}
}
```
在这个示例中,我们将字符串列表转换为一个以字符串为键、字符串长度为值的`Map`。输出结果为`{A=1, B=1, C=1}`。你可以根据你的需求修改转换的逻辑。
相关问题
list.steam.map.max空指针排除
为了避免list.steam.map.max出现空指针,可以进行以下处理:
1. 在使用list.steam.map.max之前,先判断list是否为空,如果为空则不进行操作。
2. 在创建list时,初始化list.steam.map.max的值,避免出现未初始化的情况。
3. 使用智能指针来管理list,避免手动释放内存时出现空指针。
4. 在出现空指针时,及时进行异常处理或错误提示,避免程序崩溃或出现其他错误。
例如,在使用list.steam.map.max之前,可以加上如下代码:
if(!list.empty()){
//使用list.steam.map.max进行操作
}
在创建list时,可以加上如下代码:
list.steam.map.max = 0;
使用智能指针来管理list,可以如下所示:
std::shared_ptr<std::list<int>> list_ptr(new std::list<int>());
list_ptr->steam.map.max = 0;
在出现空指针时,可以加上如下代码:
if(list == nullptr){
//发现空指针,进行异常处理或错误提示
}
steam list转map
使用Stream将List转换为Map的方法是使用Collectors的toMap方法。在toMap方法中,你需要提供两个参数,一个是用于生成Map的键的函数,另一个是用于生成Map的值的函数。例如,假设你有一个List<Person>的集合,其中Person类有id和name属性。你可以使用以下代码将List转换为Map:
Map<String, String> map = list.stream()
.collect(Collectors.toMap(Person::getId, Person::getName));
这将使用Person对象的id作为键,使用name作为对应的值,生成一个Map<String, String>。
如果在List中存在重复的键,你可以使用toMap方法的第三个参数,这是一个合并函数,用于处理重复的键。例如,如果根据id生成的Map中存在重复的键值对,你可以将重复的值组成一个集合。以下是一个示例代码:
Map<String, List<String>> map = list.stream()
.collect(Collectors.toMap(Person::getId,
p -> {
List<String> getNameList = new ArrayList<>();
getNameList.add(p.getName());
return getNameList;
},
(value1, value2) -> {
value1.addAll(value2);
return value1;
}));
这将生成一个Map<String, List<String>>,其中键是id,值是一个包含所有重复键的name的List集合。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [详解Java8新特性Stream之list转map及问题解决](https://blog.csdn.net/weixin_44864668/article/details/118275326)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
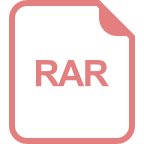












