用python实现直线随着函数图形上的某点随着角度转动
时间: 2024-10-01 08:03:11 浏览: 25
在Python中,可以使用matplotlib库来创建动态图,例如让一条直线跟随函数图像上某个点的变化而旋转。这通常通过改变直线上两个端点的角度来实现,然后结合matplotlib的事件处理机制如`FuncAnimation`。
首先,你需要定义一个函数生成图形,并在其中选择一个点。然后,你可以定义一个线段的起点和终点,这两个点会随点的位置变化而变化。下面是一个简化的示例:
```python
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.animation import FuncAnimation
# 定义一个简单的正弦曲线
def func(x):
return np.sin(x)
# 初始化动画的一些参数
fig, ax = plt.subplots()
line, = ax.plot([], [], lw=2) # 创建空线段
point, = ax.plot([], []) # 创建标记点
angle = 0 # 初始角度
x_data, y_data = [], [] # 存储点的数据
# 更新函数
def update(frame):
nonlocal angle, x_data, y_data
# 随机选取函数图像上的一个点
x = np.random.uniform(0, 2 * np.pi)
y = func(x)
# 计算新的线段端点坐标
x1, y1 = x - 1, y - 1 # 线段的一个固定端点
x2, y2 = x + 1, y + 1 # 另一端点按角度旋转
line.set_data([x1, x2], [y1, y2]) # 更新线段
point.set_data([x, x], [y, y]) # 更新标记点位置
# 更新角度
angle += 0.01 # 角度增加,每帧改变一点
rotation_matrix = np.array([[np.cos(angle), -np.sin(angle)],
[np.sin(angle), np.cos(angle)]])
new_x1, new_y1 = np.dot(rotation_matrix, [x1 - x, y1 - y]) + x
new_x2, new_y2 = np.dot(rotation_matrix, [x2 - x, y2 - y]) + x
line.set_transform(rotation_from_axes((new_x1, new_y1), (new_x2, new_y2))) # 更新旋转变换
x_data.append(x)
y_data.append(y)
return line,
# 创建动画
ani = FuncAnimation(fig, update, frames=np.arange(0, 5*np.pi, 0.01), blit=True)
plt.show()
阅读全文
相关推荐
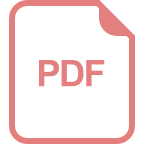
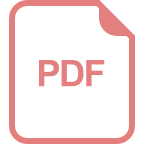
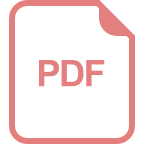
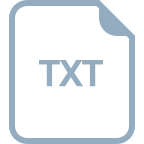
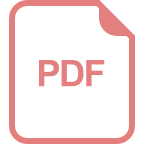
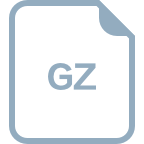
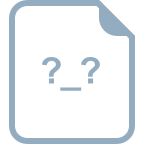
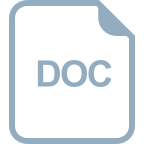
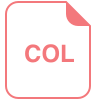
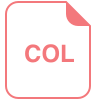
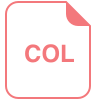


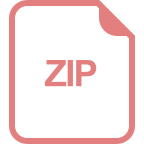
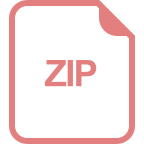
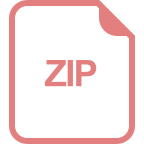