handle_integers作用
时间: 2024-10-08 09:02:26 浏览: 24
`handle_integers`通常是一个函数名,这个名称暗示它主要用于处理整数数据。它的具体功能取决于上下文,可能是用于数据处理、错误检查、算法实现等场景。它可能接收一串整数输入,对其进行解析、转换、计算、验证,甚至是格式化操作。比如,它可能在数据分析工具中用于标准化整数数据,或者在Web应用中处理用户提交的整数表单值。
举个例子:
```python
def handle_integers(integers_list):
try:
cleaned_ints = [int(num) for num in integers_list if isinstance(num, int)]
return sum(cleaned_ints)
except ValueError:
print("输入包含非整数值")
# 使用示例
input_data = ["1", "2", "three"]
result = handle_integers(input_data) # 结果将是3,忽略"three"
```
在这个例子中,`handle_integers`函数会过滤并求和输入列表中的所有整数。
相关问题
1.Write a Java program called ArithmeticT1 that takes three command-line arguments: two integers and in between an arithmetic operator (+, ‐, *, /). The program shall perform the corresponding operation on the two integers and display the result. Note that you need to deal with the nonnumeric input as operands (one of the two integers) using exception handler (try-catch statements) - the program terminates if any operand is nonnumeric, and it should display a message with the wrong operand input before exiting. Besides, the program should also handle the wrong input for operator.
下面是Java程序,用于执行两个整数之间的算术运算,并处理非数字操作数和错误输入操作符:
```java
public class ArithmeticT1 {
public static void main(String[] args) {
try {
int num1 = Integer.parseInt(args[0]);
int num2 = Integer.parseInt(args[1]);
char operator = args[2].charAt(0);
int result = 0;
switch (operator) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
result = num1 / num2;
break;
default:
throw new IllegalArgumentException("Invalid operator: " + operator);
}
System.out.println(num1 + " " + operator + " " + num2 + " = " + result);
} catch (NumberFormatException e) {
System.out.println("Error: Non-numeric operand provided.");
} catch (IllegalArgumentException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
```
该程序使用了try-catch语句来处理非数字操作数和错误输入操作符。如果非数字操作数被提供,程序将抛出NumberFormatException异常并显示错误消息。如果输入了错误的操作符,程序将抛出IllegalArgumentException异常并显示错误消息。否则,程序将执行相应的算术运算,并显示结果。
Design a java program that reads a file containing a list of integers, and calculates the average of those integers. The program should handle any exceptions that may occur during file input/output or calculation of the average, and provide appropriate error messages to the user. Additionally, the program should allow the user to specify the file name and path as a command line argument when running the program. This program takes a command line argument specifying the file name, path, and reads the file line by line using a BufferedReader. It attempts to parse each line as an integer using Integer.parseInt(), and adds it to a running sum and count. If a line is not a valid integer, it prints an error message but continues processing the rest of the file. If the file is empty or does not contain any valid integers, it prints an error message. Finally, it calculates the average and prints it to the console. The program also handles various exceptions that may occur during file input/output or calculation of the average, and prints appropriate error messages to the user.
Sure, here's a sample Java program that meets your requirements:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class AverageCalculator {
public static void main(String[] args) {
if (args.length != 1) {
System.err.println("Usage: java AverageCalculator <filename>");
System.exit(1);
}
String filename = args[0];
int sum = 0;
int count = 0;
try (BufferedReader reader = new BufferedReader(new FileReader(filename))) {
String line;
while ((line = reader.readLine()) != null) {
try {
int num = Integer.parseInt(line.trim());
sum += num;
count++;
} catch (NumberFormatException e) {
System.err.println("Invalid integer on line: " + line);
}
}
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
System.exit(1);
}
if (count == 0) {
System.err.println("No valid integers found in file.");
System.exit(1);
}
double average = (double) sum / count;
System.out.printf("Average of %d integers is %.2f\n", count, average);
}
}
```
To use this program, you would compile it using `javac AverageCalculator.java`, and then run it using `java AverageCalculator <filename>`, where `<filename>` is the name and path of the file containing the integers to be averaged. For example, if the file is called `numbers.txt` and is located in the same directory as the program, you would run `java AverageCalculator numbers.txt`.
The program first checks that the correct number of command line arguments were provided. If not, it prints an error message and exits. Otherwise, it reads in the file using a `BufferedReader`, and attempts to parse each line as an integer using `Integer.parseInt()`. If the line is not a valid integer, it prints an error message but continues processing the rest of the file. If the file is empty or does not contain any valid integers, it prints an error message and exits.
Finally, the program calculates the average by dividing the sum by the count, and prints it to the console. The program also handles various exceptions that may occur during file input/output or calculation of the average, and prints appropriate error messages to the user.
阅读全文
相关推荐
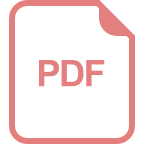
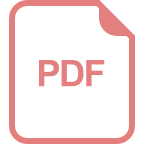
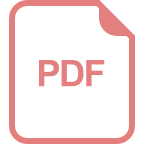
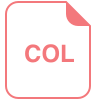




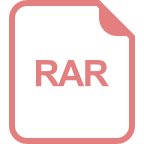
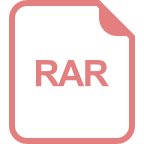
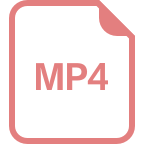
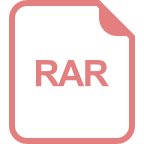
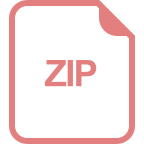