克里金插值算法 c++
时间: 2023-11-25 07:51:23 浏览: 317
以下是C++实现克里金插值的代码示例:
```c++
#include <iostream>
#include <fstream>
#include <cmath>
#include <vector>
#include <algorithm>
using namespace std;
// 定义点的结构体
struct Point {
double x, y, z;
};
// 定义距离的结构体
struct Distance {
int index;
double distance;
};
// 计算两点之间的距离
double calcDistance(Point p1, Point p2) {
return sqrt(pow(p1.x - p2.x, 2) + pow(p1.y - p2.y, 2));
}
// 计算反距离加权插值
double calcIDW(vector<Point> points, Point p, int k) {
double numerator = 0.0;
double denominator = 0.0;
vector<Distance> distances;
for (int i = 0; i < points.size(); i++) {
double distance = calcDistance(points[i], p);
distances.push_back({i, distance});
}
sort(distances.begin(), distances.end(), [](Distance d1, Distance d2) {
return d1.distance < d2.distance;
});
for (int i = 0; i < k; i++) {
int index = distances[i].index;
double distance = distances[i].distance;
numerator += points[index].z / pow(distance, 2);
denominator += 1 / pow(distance, 2);
}
return numerator / denominator;
}
// 计算最邻近点插值
double calcNN(vector<Point> points, Point p) {
double minDistance = calcDistance(points[0], p);
double z = points[0].z;
for (int i = 1; i < points.size(); i++) {
double distance = calcDistance(points[i], p);
if (distance < minDistance) {
minDistance = distance;
z = points[i].z;
}
}
return z;
}
int main() {
// 读取数据
ifstream fin("data.txt");
vector<Point> points;
double x, y, z;
while (fin >> x >> y >> z) {
points.push_back({x, y, z});
}
fin.close();
// 计算插值
Point p = {1.0, 1.0, 0.0};
int k = 3;
double idw = calcIDW(points, p, k);
double nn = calcNN(points, p);
// 输出结果
cout << "反距离加权插值:" << idw << endl;
cout << "最邻近点插值:" << nn << endl;
return 0;
}
```
阅读全文
相关推荐
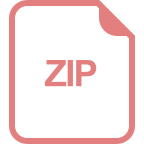
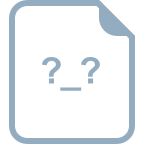
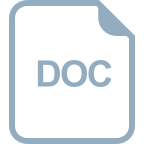
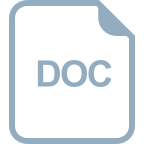
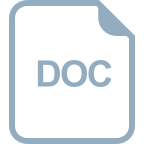



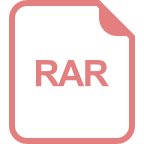
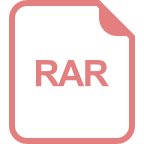
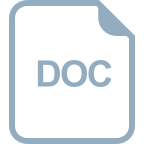




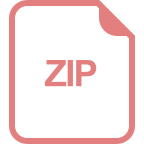
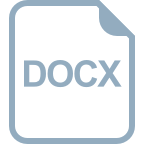