图像去噪 pyqt5 操作界面
时间: 2024-05-26 19:09:09 浏览: 102
图像去噪是一种常见的图像处理技术,可以帮助消除图像中的噪声,提高图像的质量。而PyQt5则是一种基于Python语言的GUI编程工具,可以用来设计和开发图形化界面。在PyQt5中,我们可以通过使用QImage和QPixmap等类来加载和显示图像,同时也可以使用各种滤波器来实现图像去噪。
以下是一个简单的图像去噪操作界面的示例:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QPushButton, QFileDialog
from PyQt5.QtGui import QImage, QPixmap
from PyQt5.QtCore import Qt
import cv2
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建标签和按钮
self.label = QLabel(self)
self.label.setAlignment(Qt.AlignCenter)
self.btn_open = QPushButton('打开图片', self)
self.btn_open.clicked.connect(self.open_image)
self.btn_denoise = QPushButton('去噪', self)
self.btn_denoise.clicked.connect(self.denoise_image)
# 创建布局
central_widget = QLabel(self)
layout = QVBoxLayout(central_widget)
layout.addWidget(self.label)
layout.addWidget(self.btn_open)
layout.addWidget(self.btn_denoise)
self.setCentralWidget(central_widget)
def open_image(self):
# 打开图片并显示
file_path, _ = QFileDialog.getOpenFileName(self, '打开图片', '.', 'Image Files (*.jpg *.png *.bmp)')
if file_path:
image = cv2.imread(file_path)
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
h, w, c = image.shape
bytesPerLine = c * w
q_image = QImage(image.data, w, h, bytesPerLine, QImage.Format_RGB888)
pixmap = QPixmap.fromImage(q_image)
self.label.setPixmap(pixmap)
def denoise_image(self):
# 对图片进行去噪处理
pixmap = self.label.pixmap()
if pixmap is not None:
image = pixmap.toImage()
w, h = image.width(), image.height()
ptr = image.bits()
ptr.setsize(image.byteCount())
arr = np.array(ptr).reshape(h, w, 4)[:, :, :3]
arr = cv2.fastNlMeansDenoisingColored(arr, None, 10, 10, 7, 21)
q_image = QImage(arr.data, w, h, arr.strides, QImage.Format_RGB888)
pixmap = QPixmap.fromImage(q_image)
self.label.setPixmap(pixmap)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
阅读全文
相关推荐
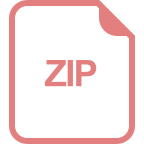
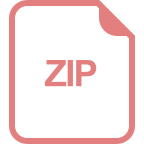
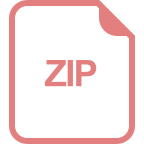
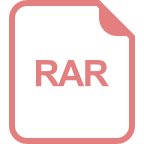
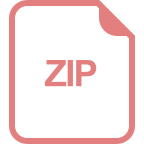
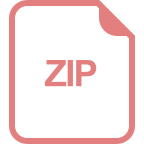
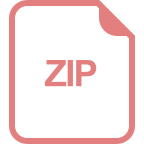
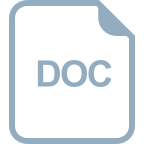
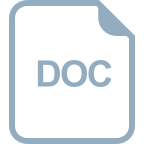
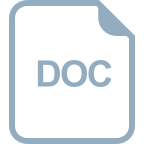
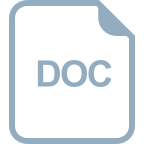
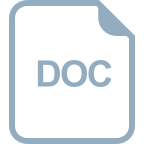
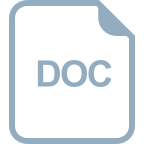
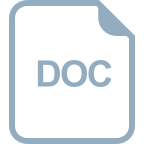
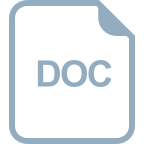
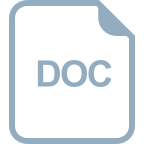