使用C++实现D435相机两个红外数据读取,并通过opencv显示
时间: 2024-12-14 16:18:54 浏览: 74
在C++中,使用Intel RealSense D435深度相机获取红外图像并通过OpenCV展示,你需要以下几个步骤:
1. **安装必要的库**:
- 包含`librealsense2`库,这是Intel RealSense SDK的一部分,用于处理相机通信。
- 安装OpenCV,用于图像处理和显示。
```sh
sudo apt-get install librealsense2 librealsense2-dev
pip install opencv-python
```
2. **设置环境变量** (如果需要):
- 配置`LD_LIBRARY_PATH`以便找到RealSense库文件。
```cpp
#include <rs2/hardware_device.hpp>
#include <rs2/types.h>
```
3. **初始化相机**:
创建`rs2::context`对象来管理设备连接。
```cpp
rs2::context ctx;
auto dev = ctx.query_devices().front(); // 获取第一个可用设备
```
4. **启动红外序列**:
选择红外相机的数据流。
```cpp
rs2::config config;
config.enable_stream(rs2::stream::infrared, 1);
auto pipeline = rs2::pipeline();
pipeline.start(config);
```
5. **读取红外帧**:
使用循环从管道中抓取红外帧。
```cpp
rs2::frame frame;
while (true) {
auto wait_result = pipeline.wait_for_frame(1000);
if (wait_result == rs2::pipeline_status::frame_data_available) {
if (frame = pipeline.get_frame()) {
break; // 打断循环,因为我们已经获取了帧
}
} else if (wait_result == rs2::pipeline_status::no_frames) {
continue; // 如果没有新帧,继续等待
} else {
throw std::runtime_error("Error waiting for frames");
}
}
```
6. **转换到OpenCV兼容格式**:
将IR帧转换为OpenCV Mat对象。
```cpp
cv::Mat ir_image(frame.get_data(), frame.get_format(), cv::Size(frame.get_width(), frame.get_height()));
```
7. **显示图像**:
使用OpenCV函数显示图像。
```cpp
cv::imshow("Infrared Image", ir_image);
cv::waitKey(1); // 等待用户按键
```
8. **关闭资源**:
关闭摄像头和窗口。
```cpp
pipeline.stop();
ctx.release();
cv::destroyAllWindows();
```
阅读全文
相关推荐







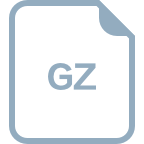










