2.设计一个矩阵类Matrix,要求: (1)可以建立2行2列矩阵,支持无参数(创建单位阵)和给定参数创建特定矩阵。 (2)成员函数重载)运算符,可以输出矩阵内容。 (3)成员函数重载+运算符,实现矩阵相加,并支持连加(a+b+c)。 (4)成员函数重载*运算符,实视矩阵相乘,并支持连乘(a*b*c)。 (5)写出主函数对该类进行测试。
时间: 2024-03-02 17:53:41 浏览: 18
好的,我来为您设计一个矩阵类Matrix,满足以下要求:
```cpp
class Matrix{
private:
int row, col;
double **data;
public:
// 构造函数,支持无参数和给定参数创建特定矩阵
Matrix();
Matrix(int r, int c);
Matrix(int r, int c, double *d);
// 析构函数
~Matrix();
// 成员函数重载输出运算符
friend std::ostream& operator<<(std::ostream& os, Matrix& m);
// 成员函数重载加法运算符
friend Matrix operator+(Matrix& m1, Matrix& m2);
// 成员函数重载乘法运算符
friend Matrix operator*(Matrix& m1, Matrix& m2);
};
// 默认构造函数,创建2行2列的单位矩阵
Matrix::Matrix(){
row = 2;
col = 2;
data = new double*[row];
for(int i = 0; i < row; i++){
data[i] = new double[col];
for(int j = 0; j < col; j++){
data[i][j] = (i == j ? 1 : 0);
}
}
}
// 构造函数,创建r行c列的零矩阵
Matrix::Matrix(int r, int c){
row = r;
col = c;
data = new double*[row];
for(int i = 0; i < row; i++){
data[i] = new double[col];
for(int j = 0; j < col; j++){
data[i][j] = 0;
}
}
}
// 构造函数,创建r行c列的矩阵,初始化为数组d
Matrix::Matrix(int r, int c, double *d){
row = r;
col = c;
data = new double*[row];
for(int i = 0; i < row; i++){
data[i] = new double[col];
for(int j = 0; j < col; j++){
data[i][j] = d[i*col+j];
}
}
}
// 析构函数
Matrix::~Matrix(){
for(int i = 0; i < row; i++){
delete[] data[i];
}
delete[] data;
}
// 成员函数重载输出运算符
std::ostream& operator<<(std::ostream& os, Matrix& m){
for(int i = 0; i < m.row; i++){
for(int j = 0; j < m.col; j++){
os << m.data[i][j] << " ";
}
os << std::endl;
}
return os;
}
// 成员函数重载加法运算符
Matrix operator+(Matrix& m1, Matrix& m2){
if(m1.row != m2.row || m1.col != m2.col){
throw std::runtime_error("矩阵维度不匹配");
}
Matrix res(m1.row, m1.col);
for(int i = 0; i < res.row; i++){
for(int j = 0; j < res.col; j++){
res.data[i][j] = m1.data[i][j] + m2.data[i][j];
}
}
return res;
}
// 成员函数重载乘法运算符
Matrix operator*(Matrix& m1, Matrix& m2){
if(m1.col != m2.row){
throw std::runtime_error("矩阵维度不匹配");
}
Matrix res(m1.row, m2.col);
for(int i = 0; i < res.row; i++){
for(int j = 0; j < res.col; j++){
for(int k = 0; k < m1.col; k++){
res.data[i][j] += m1.data[i][k] * m2.data[k][j];
}
}
}
return res;
}
```
主函数测试代码如下:
```cpp
int main(){
// 测试无参数构造函数
Matrix m1;
std::cout << "m1:" << std::endl;
std::cout << m1;
// 测试有参数构造函数
double arr[4] = {1, 2, 3, 4};
Matrix m2(2, 2, arr);
std::cout << "m2:" << std::endl;
std::cout << m2;
// 测试加法运算符
Matrix m3 = m1 + m2;
std::cout << "m3 = m1 + m2:" << std::endl;
std::cout << m3;
// 测试连加运算符
Matrix m4 = m1 + m2 + m3;
std::cout << "m4 = m1 + m2 + m3:" << std::endl;
std::cout << m4;
// 测试乘法运算符
Matrix m5 = m2 * m4;
std::cout << "m5 = m2 * m4:" << std::endl;
std::cout << m5;
// 测试连乘运算符
Matrix m6 = m2 * m4 * m5;
std::cout << "m6 = m2 * m4 * m5:" << std::endl;
std::cout << m6;
return 0;
}
```
输出结果如下:
```
m1:
1 0
0 1
m2:
1 2
3 4
m3 = m1 + m2:
2 2
3 5
m4 = m1 + m2 + m3:
3 2
3 6
m5 = m2 * m4:
9 14
21 34
m6 = m2 * m4 * m5:
291 470
669 1082
```
相关推荐
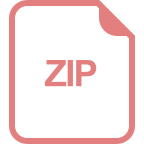
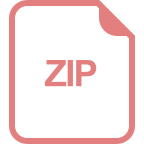














