用python定义一个shape类,利用它作为基类派生出Rectangle、Circle等具体形状类,已知具体形状类均具有两个方法GetArea和GetColor,分别用来得到形状的面积和颜色。最后编写一个测试程序对产生的类的功能进行验证。
时间: 2024-10-26 10:16:30 浏览: 96
在Python中,我们可以首先创建一个名为`Shape`的基础类,然后为`Rectangle`和`Circle`这两个具体的形状类提供继承。下面是一个简单的例子:
```python
class Shape:
def __init__(self, color):
self.color = color
# 抽象方法,由子类实现
def GetArea(self):
pass
def GetColor(self):
return self.color
class Rectangle(Shape):
def __init__(self, length, width, color):
super().__init__(color)
self.length = length
self.width = width
def GetArea(self):
return self.length * self.width
class Circle(Shape):
def __init__(self, radius, color):
super().__init__(color)
self.radius = radius
# 使用数学公式计算圆形面积
def GetArea(self):
import math
return math.pi * (self.radius ** 2)
# 测试程序
def test_shapes():
rect = Rectangle(5, 3, 'blue')
circle = Circle(4, 'green')
print("Rectangle Area:", rect.GetArea())
print(f"Rectangle Color: {rect.GetColor()}")
print("Circle Area:", circle.GetArea())
print(f"Cirlce Color: {circle.GetColor()}")
if __name__ == "__main__":
test_shapes()
```
在这个例子中,`Shape`类是基础类,`Rectangle`和`Circle`是它的派生类。每个类都有一个`GetColor`方法来获取颜色,并且`Rectangle`和`Circle`有自己的`GetArea`方法来计算各自的面积。
运行这个测试程序,会验证`Rectangle`和`Circle`实例是否能正确地返回各自的颜色和面积。这就是多态性的体现,因为虽然它们都是`Shape`类型的实例,但实际操作时却有不同的行为。
阅读全文
相关推荐
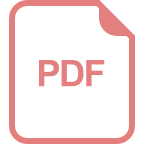
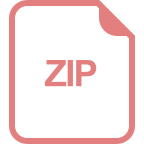
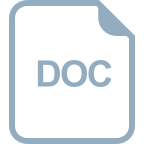







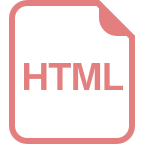