定义一个Shape类,利用它作为基类派生出Rectangle、Circle等具体形状类,已知具体形状类均具有两个方法GetArea和GetColor,分别用来得到形状的面积和颜色。最后编写一个测试程序对产生的类的功能进行验证。
时间: 2023-11-13 15:10:27 浏览: 30
好的,这是一个关于面向对象编程的问题,属于技术类问题。我可以回答你。 首先,定义一个Shape类,如下:
```python
class Shape:
def __init__(self, color):
self.color = color
def GetColor(self):
return self.color
def GetArea(self):
pass
```
其中,初始化函数__init__接收一个参数color作为形状的颜色,GetColor方法返回形状的颜色,GetArea方法暂时为空,因为不同形状的计算面积方式不同,需要由具体形状类实现。
接下来,我们可以通过继承Shape类,派生出Rectangle和Circle类,如下:
```python
class Rectangle(Shape):
def __init__(self, color, width, height):
super().__init__(color)
self.width = width
self.height = height
def GetArea(self):
return self.width * self.height
class Circle(Shape):
def __init__(self, color, radius):
super().__init__(color)
self.radius = radius
def GetArea(self):
return 3.14 * self.radius ** 2
```
具体形状类继承Shape类,并实现自己的GetArea方法来计算面积。Rectangle类接收width和height两个参数,Circle类接收radius参数。
最后,我们可以编写一个测试程序验证类的功能:
```python
r = Rectangle('red', 3, 4)
c = Circle('blue', 5)
print(r.GetColor())
print(r.GetArea())
print(c.GetColor())
print(c.GetArea())
```
输出结果为:
```
red
12
blue
78.5
```
其中,r实例化了一个红色的矩形,面积为12;c实例化了一个蓝色的圆形,面积为78.5。
相关推荐
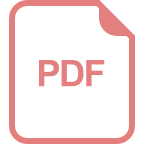
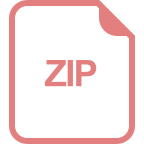
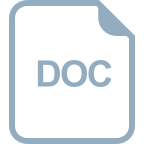






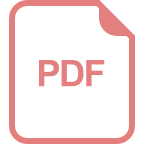
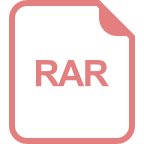
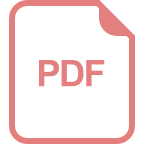
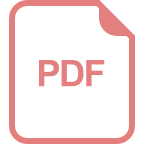
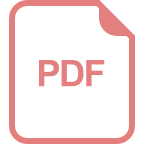
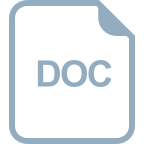