定义一个shape类,利用它作为基类派生出Rectangle、Circle等具体形状类,已知具体形状类均具有两个方法GetArea和GetColor,分别用来得到形状的面积和颜色。最后编写一个测试程序对产生的类的功能进行验证。
时间: 2024-10-26 08:16:29 浏览: 83
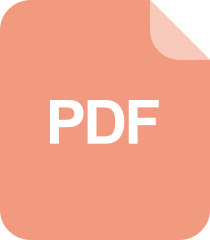
详解C++中基类与派生类的转换以及虚基类
首先,我们定义一个基础Shape类,包含虚方法GetArea和GetColor:
```cpp
class Shape {
public:
virtual double GetArea() const = 0; // 虚函数,用于计算面积
virtual std::string GetColor() const = 0; // 虚函数,获取颜色
protected:
Shape() {}
};
```
然后,我们可以为Rectangle和Circle这两个具体形状类提供各自的实现:
```cpp
class Rectangle : public Shape {
private:
double width;
double height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
double GetArea() const override { return width * height; }
std::string GetColor() const override { return "Red"; } // 示例颜色,实际应用可根据需要设置
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double GetArea() const override { return M_PI * radius * radius; }
std::string GetColor() const override { return "Blue"; } // 示例颜色,同样可自定义
};
```
接下来,我们编写一个测试程序来验证这些类的功能:
```cpp
#include <iostream>
int main() {
Shape* shape1 = new Rectangle(5, 4);
Shape* shape2 = new Circle(3);
std::cout << "Rectangle area: " << shape1->GetArea() << ", Color: " << shape1->GetColor() << std::endl;
std::cout << "Circle area: " << shape2->GetArea() << ", Color: " << shape2->GetColor() << std::endl;
delete shape1;
delete shape2;
return 0;
}
```
在这个测试程序中,我们创建了Rectangle和Circle的对象,并通过Shape指针调用它们的GetArea和GetColor方法。这展示了多态性的效果,因为虽然我们直接操作的是Shape类型的指针,但是实际上调用了各自子类的具体实现。
阅读全文
相关推荐
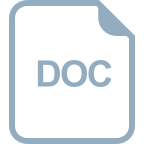
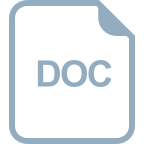








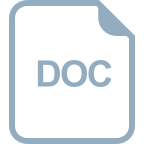
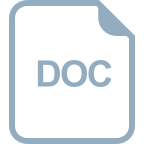
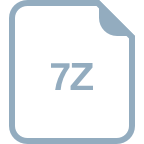
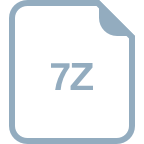
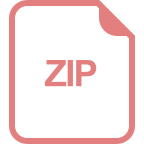