C# 如何在代码中循环WPF窗口中的控件
时间: 2024-11-29 22:37:47 浏览: 10
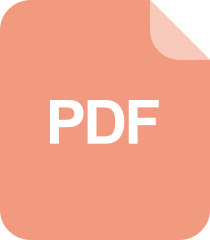
C# 使用WPF 用MediaElement控件实现视频循环播放
在C# WPF中,如果你想要遍历窗体(Window)中的控件,你可以使用`VisualTreeHelper`类来递归地访问所有子元素。这里是一个简单的示例:
```csharp
using System.Windows.Media;
using System.Windows.Media.VisualTree;
public static void TraverseControls(Window window)
{
if (window != null)
{
for (DependencyObject child = VisualTreeHelper.GetChildrenCount(window) > 0 ? VisualTreeHelper.GetChild(window, 0) : null;
child != null;
child = VisualTreeHelper.GetNextSibling(child))
{
// 遍历控件并处理每个控件
FrameworkElement frameworkChild = child as FrameworkElement;
if (frameworkChild != null)
{
Console.WriteLine($"控件名称: {frameworkChild.Name}, 类型: {frameworkChild.GetType().Name}");
// 这里可以添加对控件的操作,比如访问属性、响应事件等
}
}
// 递归遍历子窗口(如果窗体有嵌套的窗口)
foreach (Window subWindow in GetWindowsRecursively(window))
{
TraverseControls(subWindow);
}
}
}
private static IEnumerable<Window> GetWindowsRecursively(DependencyObject obj)
{
for (DependencyObject child = VisualTreeHelper.GetChildrenCount(obj) > 0 ? VisualTreeHelper.GetChild(obj, 0) : null;
child != null;
child = VisualTreeHelper.GetNextSibling(child))
{
FrameworkElement element = child as FrameworkElement;
if (element != null && element is Window)
{
yield return (Window)element;
}
else if (child is DependencyObject dependencyObj && dependencyObj.IsArrangeValid)
{
foreach (Window window in GetWindowsRecursively(dependencyObj))
{
yield return window;
}
}
}
}
```
在这个例子中,`TraverseControls`函数会从给定的窗口开始,逐级深入子树,检查每一个`FrameworkElement`类型的节点(如控件)。如果你想针对特定类型或特性进行操作,可以在`if (frameworkChild != null)`条件内添加相应的代码。
阅读全文
相关推荐
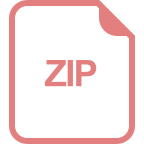
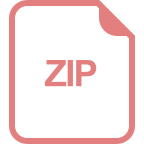
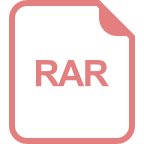
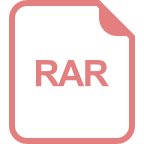
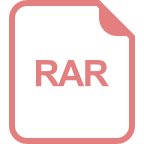
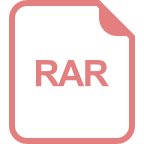
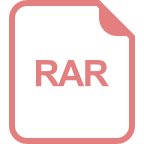
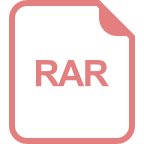
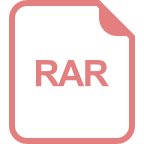
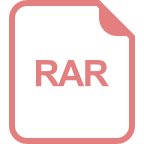
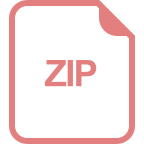
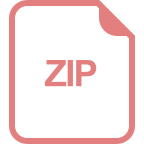
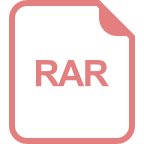
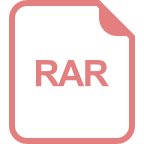
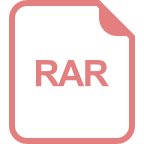
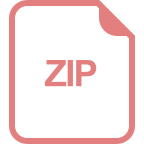
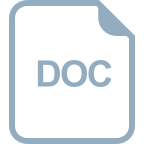
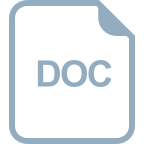