用C语言写双线程代码
时间: 2024-09-17 19:04:40 浏览: 39
在C语言中,由于原生库提供的支持有限,直接编写复杂的多线程程序可能需要依赖于POSIX线程库(pthreads)。以下是一个简单的双线程示例,创建了两个线程,每个线程打印数字:
```c
#include <stdio.h>
#include <pthread.h>
// 线程函数原型
void* print_numbers(void* arg);
int main() {
pthread_t thread1, thread2; // 创建线程的变量
int count = 5; // 每个线程打印的次数
// 创建并启动线程1
if (pthread_create(&thread1, NULL, print_numbers, &count) != 0) {
perror("Thread creation failed");
return 1;
}
// 创建并启动线程2
if (pthread_create(&thread2, NULL, print_numbers, &count) != 0) {
perror("Thread creation failed");
return 1;
}
// 等待两个线程结束
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
printf("Both threads finished.\n");
return 0;
}
// 线程函数,接收一个整数参数表示循环次数
void* print_numbers(void* num_ptr) {
int count = *((int*)num_ptr); // 解引用指针获取实际值
for (int i = 0; i < count; i++) {
printf("Thread %d: %d\n", pthread_self(), i);
// 这里可以替换为线程的实际任务
}
return NULL;
}
```
阅读全文
相关推荐
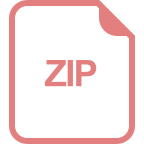
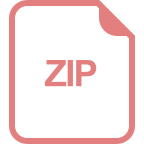
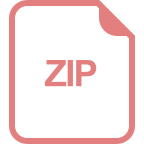


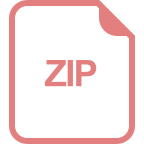
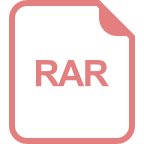
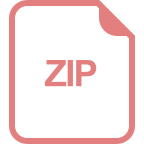
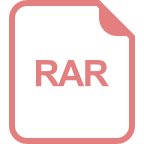
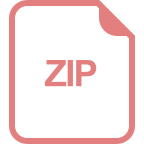
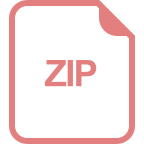
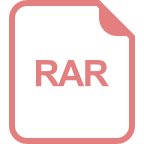
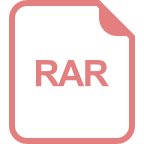
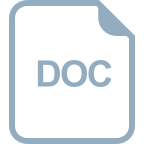
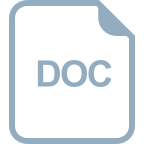
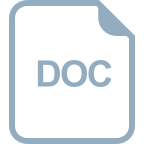
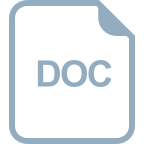
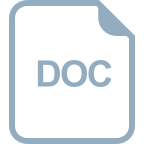
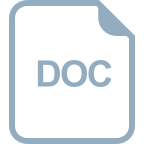