def encrypt_bytes(data,key): #加密 e_data = [] for d in data: e = modpow(d, key[0], key[1])#modpow函数,它用来计算公式b^e % n = r #如果是加密过程,那么b 是明文,(n,e)为公钥, r 为密文。 #如果是解密过程,那么b 是密文,(n,d)为私钥, r 为明文。 e_data.append(e)#append用于列表后添加新的对象,在这里是完成e_data的赋值 return e_data decrypt_bytes = encrypt_bytes什么意思
时间: 2024-03-18 10:43:11 浏览: 50
这段代码定义了两个函数 `encrypt_bytes()` 和 `decrypt_bytes()`,它们用于实现 RSA 加密和解密。
具体来说,`encrypt_bytes()` 函数接收两个参数,一个是需要加密的明文数据 `data`,另一个是包含公钥信息的元组 `key`。在函数内部,它通过遍历 `data` 中的每个字节,使用公钥信息对其进行 RSA 加密,并将加密后的密文依次添加到一个列表 `e_data` 中,并最终返回该列表。
`decrypt_bytes()` 函数则与 `encrypt_bytes()` 函数完全相同,只不过它的作用是进行 RSA 解密,因此将其实现定义为 `decrypt_bytes = encrypt_bytes`,即将解密函数定义为与加密函数完全相同的函数。
需要说明的是,这里的 RSA 加密算法使用了快速幂算法来计算幂的值,具体实现在 `modpow()` 函数中,该函数用来计算公式 b^e % n = r 的值,其中 b 表示明文或密文,e 表示加密或解密时使用的指数,n 和 (n, e) 或 (n, d) 表示 RSA 加密或解密时使用的模数和公钥或私钥。
相关问题
注释下列代码from Crypto import Random from Crypto.PublicKey import RSA random_generator = Random.new().read rsa = RSA.generate(2048, random_generator) # 生成私钥 private_key = rsa.exportKey() #导出私钥 print(private_key.decode('utf-8')) #以utf-8格式解码并打印私钥 # 生成公钥 public_key = rsa.publickey().exportKey() #导出私钥 print(public_key.decode('utf-8')) #以utf-8格式解码并打印公钥 with open('rsa_private_key.pem', 'wb')as f: f.write(private_key) #打开私钥文件并进行写操作 with open('rsa_public_key.pem', 'wb')as f: f.write(public_key) #打开公钥文件并进行写操作 import base64 #导入base64编码 from Crypto.PublicKey import RSA #导入PulbicKey库 from Crypto.Hash import SHA #导入Hash库 from Crypto.Signature import PKCS1_v1_5 as PKCS1_signature #导入Signature库 from Crypto.Cipher import PKCS1_v1_5 as PKCS1_cipher #导入Cipher库 # def get_key(key_file): with open(key_file) as f: data = f.read() key = RSA.importKey(data) return key def encrypt_data(msg): public_key = get_key('rsa_public_key.pem') cipher = PKCS1_cipher.new(public_key) encrypt_text = base64.b64encode(cipher.encrypt(bytes(msg.encode("utf8")))) return encrypt_text.decode('utf-8') def decrypt_data(encrypt_msg): private_key = get_key('rsa_private_key.pem') cipher = PKCS1_cipher.new(private_key) back_text = cipher.decrypt(base64.b64decode(encrypt_msg), 0) return back_text.decode('utf-8') def test_encrypt_decrypt(): msg = "coolpython.net" encrypt_text = encrypt_data(msg) decrypt_text = decrypt_data(encrypt_text) print(msg == decrypt_text) test_encrypt_decrypt() # Tru
这段代码使用了Python的Crypto库,引入了两个模块,Random和RSA。
其中,Random模块用于生成随机数生成器,通过调用new()方法产生随机数据源。RSA模块则用于生成指定位数的RSA加密算法公钥和私钥。
在代码中,使用了2048位的密钥长度作为参数传入生成RSA密钥对的方法中,并将随机数据源传入random_generator参数中。最终,将生成的RSA密钥对存储在变量rsa中。
def xor_encrypt(file_path, key): # 打开文件以二进制形式读取数据 with open(file_path, 'rb') as file: # 读取文件数据 data = file.read() # 将每个字节与密钥进行异或加密 encrypted_data = bytearray() for byte in data: encrypted_byte = byte ^ key encrypted_data.append(encrypted_byte) # 返回加密后的数据 return encrypted_data优化
这段代码已经比较简洁明了了,但还是有些小优化可以进行:
1. 可以使用`with open() as`语句来自动关闭文件,不需要手动调用`file.close()`方法。
2. 可以使用列表推导式来替代循环,使代码更简洁。具体实现如下:
```python
def xor_encrypt(file_path, key):
# 打开文件以二进制形式读取数据
with open(file_path, 'rb') as file:
# 读取文件数据
data = file.read()
# 将每个字节与密钥进行异或加密
encrypted_data = [byte ^ key for byte in data]
# 返回加密后的数据
return bytes(encrypted_data)
```
这样代码更简洁易懂了。同时,由于使用了列表推导式,函数返回的是一个`bytes`类型,而不是`bytearray`类型。
阅读全文
相关推荐
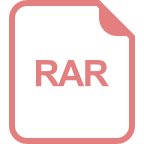
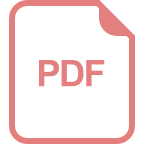

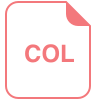
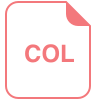
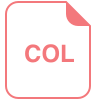
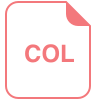
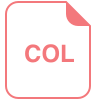
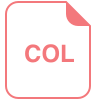
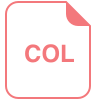
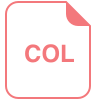
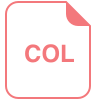
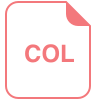
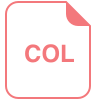
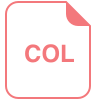
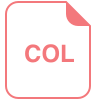