使用python 爬取网页标题并设为文本文档标题
时间: 2023-12-29 08:06:14 浏览: 21
可以使用Python中的requests库和BeautifulSoup库来爬取网页标题,并将其设置为文本文档标题。
首先,需要安装requests库和BeautifulSoup库。可以使用以下命令进行安装:
```
pip install requests
pip install beautifulsoup4
```
然后,可以使用以下代码来实现:
```python
import requests
from bs4 import BeautifulSoup
# 发送请求
url = 'https://www.example.com'
response = requests.get(url)
# 解析网页内容
soup = BeautifulSoup(response.text, 'html.parser')
# 获取网页标题
title = soup.title.string
# 创建文本文档并将标题设置为文件名
with open(title + '.txt', 'w') as f:
f.write('This is ' + title + ' document')
```
这段代码先发送请求获取网页内容,然后使用BeautifulSoup库解析网页内容。接着,获取网页标题并将其赋值给变量title。最后,使用open函数创建一个文本文档,并将标题设置为文件名。将文本写入文件中,完成操作。
相关问题
python爬取数据教程_python爬虫爬取网页数据并解析数据
好的,下面是一个简单的Python爬虫爬取网页数据并解析数据的教程:
1. 确定目标网站和要爬取的信息
首先,需要确定要爬取的网站和要提取的信息。可以使用Python的requests库向网站发送HTTP请求获取HTML源代码,并使用BeautifulSoup库解析HTML文档获取目标数据。
例如,我们要爬取CSDN博客的文章标题和链接,可以先打开CSDN博客主页,右键查看网页源代码,找到文章标题和链接所在的HTML标签。
2. 发送HTTP请求获取HTML源代码
接下来,使用Python的requests库向网站发送HTTP请求,获取HTML源代码。
```
import requests
url = 'https://blog.csdn.net/'
response = requests.get(url)
html = response.text
```
3. 解析HTML文档获取目标数据
使用BeautifulSoup库解析HTML文档,获取目标数据。
```
from bs4 import BeautifulSoup
soup = BeautifulSoup(html, 'html.parser')
titles = soup.find_all('div', class_='title')
for title in titles:
link = title.find('a').get('href')
title_text = title.find('a').text.strip()
print(title_text, link)
```
上述代码中,通过`find_all`方法找到所有class属性为"title"的div标签,然后在每个div标签中找到第一个a标签,获取链接和标题文本。
4. 完整代码
```
import requests
from bs4 import BeautifulSoup
url = 'https://blog.csdn.net/'
response = requests.get(url)
html = response.text
soup = BeautifulSoup(html, 'html.parser')
titles = soup.find_all('div', class_='title')
for title in titles:
link = title.find('a').get('href')
title_text = title.find('a').text.strip()
print(title_text, link)
```
以上就是一个简单的Python爬虫爬取网页数据并解析数据的教程。需要注意的是,在爬取网站数据时要遵守网站的爬虫协议,避免被网站封禁IP。
使用python爬取微博热门新闻并且输出到一个文本文档里
以下是使用Python爬取微博热门新闻并输出到文本文档的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 微博热搜的URL
url = "https://s.weibo.com/top/summary?cate=realtimehot"
# 发送HTTP请求并获取响应内容
response = requests.get(url)
html = response.text
# 使用BeautifulSoup解析HTML
soup = BeautifulSoup(html, "html.parser")
# 获取热门新闻的列表
news_list = soup.select(".td-02 a")
# 打开一个文本文件用于写入热门新闻
with open("weibo_hot_news.txt", "w", encoding="utf-8") as f:
# 遍历热门新闻列表,依次写入文件
for news in news_list:
news_title = news.get_text(strip=True)
f.write(news_title + "\n")
```
以上代码会爬取微博热搜的页面,获取热门新闻的标题,并将其写入一个名为"weibo_hot_news.txt"的文本文件中。你可以修改代码来适应你所需的输出格式。
相关推荐
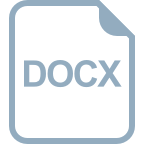
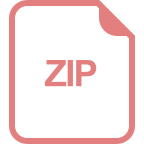
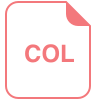
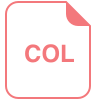
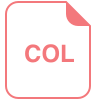
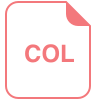
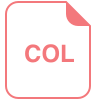







