使用pytqt5和python3语言,左右给动态旋转的漩涡彩虹,颜色要不断自动变幻,自动旋转,可以点击按钮控制顺时针和逆时针旋转
时间: 2024-03-09 10:49:37 浏览: 67
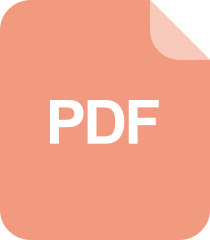
颜色旋转旋转
好的,这是一个比较复杂的需求,需要使用PyQt5的QPainter和QTimer类来实现。以下是一个简单的示例代码,可以让你开始实现这个功能:
```python
import sys
from PyQt5.QtCore import Qt, QTimer
from PyQt5.QtGui import QColor, QPainter
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton
class RainbowWidget(QWidget):
def __init__(self):
super().__init__()
# 初始化变量
self.angle = 0
self.clockwise = True
self.colors = [Qt.red, Qt.yellow, Qt.green, Qt.cyan, Qt.blue, Qt.magenta]
# 创建按钮
self.button = QPushButton("反转方向", self)
self.button.setGeometry(10, 10, 100, 30)
self.button.clicked.connect(self.reverse_direction)
# 创建定时器
self.timer = QTimer(self)
self.timer.timeout.connect(self.update)
self.timer.start(50)
def paintEvent(self, event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing)
# 旋转坐标系
painter.translate(self.width() / 2, self.height() / 2)
painter.rotate(self.angle)
# 绘制彩虹
for i in range(6):
painter.setBrush(QColor(self.colors[i]))
painter.drawEllipse(-100 + i * 20, -100 + i * 20, 200 - i * 40, 200 - i * 40)
# 更新角度
if self.clockwise:
self.angle += 1
else:
self.angle -= 1
def reverse_direction(self):
self.clockwise = not self.clockwise
def update_colors(self):
# 更新颜色
self.colors.append(self.colors.pop(0))
def update(self):
self.update_colors()
self.update()
if __name__ == '__main__':
app = QApplication(sys.argv)
widget = RainbowWidget()
widget.resize(400, 400)
widget.show()
sys.exit(app.exec_())
```
这个示例代码实现了一个旋转的彩虹漩涡,颜色不断变化,并且可以通过按钮控制顺时针和逆时针旋转。你可以根据自己的需求对代码进行修改和优化。
阅读全文
相关推荐
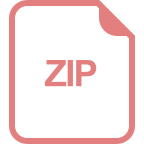
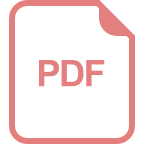
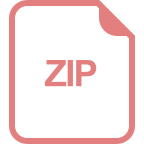
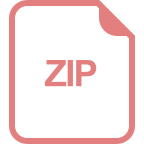
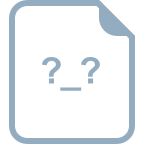
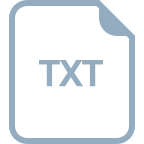
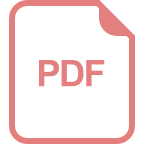
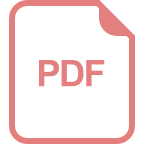
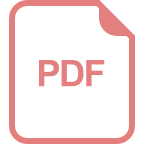
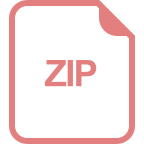
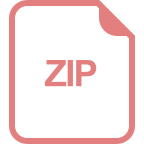
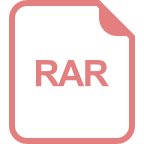
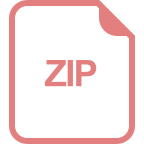
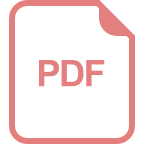
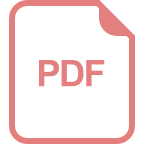