编程编写银行家算法模拟程序
时间: 2023-11-18 14:04:10 浏览: 84
银行家算法是操作系统中避免死锁的典型算法,下面是一个简单的Python实现:
```python
# 定义银行家算法类
class BankerAlgorithm:
def __init__(self, available, max_need, allocation):
self.available = available # 可用资源
self.max_need = max_need # 进程最大需求
self.allocation = allocation # 进程已分配资源
self.need = [] # 进程还需要资源
self.work = available # 工作向量
self.finish = [False] * len(allocation) # 进程是否完成标志
self.safe_sequence = [] # 安全序列
# 计算进程还需要的资源
for i in range(len(max_need)):
self.need.append([max_need[i][j] - allocation[i][j] for j in range(len(max_need[i]))])
# 判断是否存在安全序列
def is_safe(self):
while False in self.finish:
flag = False
for i in range(len(self.need)):
if not self.finish[i] and self.need[i] <= self.work:
self.work = [self.work[j] + self.allocation[i][j] for j in range(len(self.work))]
self.finish[i] = True
self.safe_sequence.append(i)
flag = True
if not flag:
return False
return True
# 分配资源
def allocate(self, process, request):
if request <= self.need[process] and request <= self.available:
self.available = [self.available[i] - request[i] for i in range(len(request))]
self.allocation[process] = [self.allocation[process][i] + request[i] for i in range(len(request))]
self.need[process] = [self.need[process][i] - request[i] for i in range(len(request))]
if self.is_safe():
return True
else:
self.available = [self.available[i] + request[i] for i in range(len(request))]
self.allocation[process] = [self.allocation[process][i] - request[i] for i in range(len(request))]
self.need[process] = [self.need[process][i] + request[i] for i in range(len(request))]
return False
else:
return False
# 显示当前时刻各进程的资源分配情况
def show_allocation(self):
print("Allocation:")
for i in range(len(self.allocation)):
print("Process", i, ":", self.allocation[i])
print("")
# 将有关请求和相应决定的列表输出到一个文件中
def output_to_file(self, filename):
with open(filename, "w") as f:
f.write("Allocation:\n")
for i in range(len(self.allocation)):
f.write("Process " + str(i) + ": " + str(self.allocation[i]) + "\n")
f.write("\n")
f.write("Max Need:\n")
for i in range(len(self.max_need)):
f.write("Process " + str(i) + ": " + str(self.max_need[i]) + "\n")
f.write("\n")
f.write("Need:\n")
for i in range(len(self.need)):
f.write("Process " + str(i) + ": " + str(self.need[i]) + "\n")
f.write("\n")
f.write("Available:\n")
f.write(str(self.available) + "\n")
f.write("\n")
f.write("Safe Sequence:\n")
f.write(str(self.safe_sequence) + "\n")
```
使用示例:
```python
# 测试
if __name__ == "__main__":
available = [3, 3, 2]
max_need = [[7, 5, 3], [3, 2, 2], [9, 0, 2], [2, 2, 2], [4, 3, 3]]
allocation = [[0, 1, 0], [2, 0, 0], [3, 0, 2], [2, 1, 1], [0, 0, 2]]
banker = BankerAlgorithm(available, max_need, allocation)
banker.show_allocation()
banker.output_to_file("output.txt")
# 请求资源
process = 1
request = [1, 0, 2]
if banker.allocate(process, request):
print("Allocate success!")
banker.show_allocation()
banker.output_to_file("output.txt")
else:
print("Allocate failed!")
```
阅读全文
相关推荐
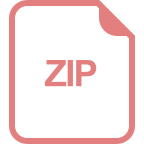
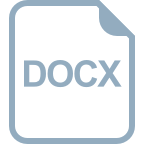
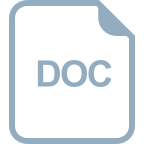








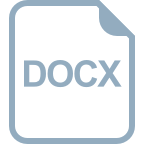
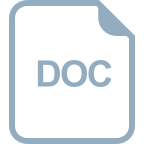
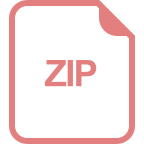
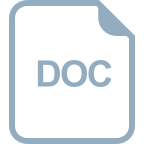
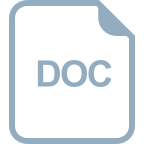
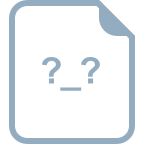
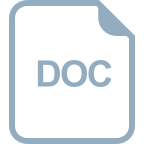
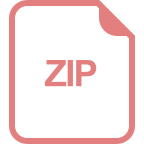