class Super { public int getLength() { return 4; } } public class Child extends Super { public long getLength() { return 5; } public static void main(String[] args) { Super sub = new Child(); System.out.print(sub.getLength()); } }
时间: 2024-02-15 17:29:09 浏览: 166
在这段代码中,输出结果为5。由于Child类继承了Super类,并重写了getLength()方法,使得子类中的getLength()方法返回类型为long,而父类中的getLength()方法返回类型为int。当子类实例化后,调用getLength()方法时,会优先调用子类中的getLength()方法,输出结果为5。这是因为Java中的方法重写是基于运行时动态绑定的,即子类方法会覆盖父类方法,调用的方法取决于实例化对象的类型。
相关问题
class Super { public int getLength() { return 4; } } public class Sub extends Super { public long getLength() { return 5; } public static void main (String[]args) { Super sooper = new Super (); Super sub = new Sub(); System.out.printIn(sooper.getLength()+ “,” + sub.getLength() }; }
这段代码有语法错误,应该将最后一行的 "}" 改为 ")",并将 printIn 改为 println。另外,在 Sub 类中,getLength 方法的返回值类型应该与父类的 getLength 方法的返回值类型一致,即都为 int 或都为 long。如果返回值类型不一致,会编译出错。如果将 Sub 类中的 getLength 方法的返回值类型改为 int,则输出结果为 "4,4";如果将其改为 long,则输出结果为 "4,5"。这是因为在 main 方法中,sooper.getLength() 调用的是 Super 类中的 getLength 方法,其返回值为 4;而 sub.getLength() 调用的是 Sub 类中的 getLength 方法,其返回值为 5。
解释代码public class BookShelf implements Aggregate { private Book[] books; private int last = 0; public BookShelf(int maxsize) { this.books = new Book[maxsize]; } public Book getBookAt(int index) { return books[index]; } public void appendBook(Book book) { this.books[last] = book; last++; } public int getLength() { return last; }
这是一个书架类的代码,实现了 Aggregate 接口。它包含了一个 Book 类型的数组 books,以及一个整型变量 last,用于记录书架上书的数量。构造函数 BookShelf(int maxsize) 用于初始化书架的最大容量。方法 getBookAt(int index) 用于获取书架上指定位置的书,方法 appendBook(Book book) 用于向书架上添加一本书,方法 getLength() 用于获取书架上的书的数量。
阅读全文
相关推荐
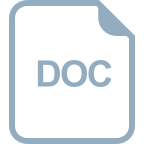
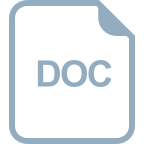
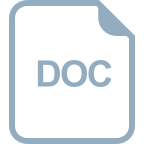













