#include<iostream> using namespace std; class Point { private: int x; int y; public: Point(int xx,int yy):x(xx),y(yy) {} int getX() { return x; } int getY() { return y; } }; class Line { private: float length; Point p1,p2; public: Line(Point a,Point b):p1(a),p2(b) {} float getLength() { return length; } length=sqrt((p1.getX()-p2.getX())*(p1.getX()-p2.getX())+(p1.getY()-p2.getY())*(p1.getY()-p2.getY())); }; int main(){ Point p1(4,4),p2(2,2); Line L(p1,p2); cout<<L.getLength(); }
时间: 2024-04-19 18:27:14 浏览: 120
你提供的代码存在一些语法错误。在Line类中,你需要在构造函数外定义length的计算语句,并且在getLength函数中返回计算出的length值。以下是修改后的代码:
```cpp
#include<iostream>
#include<cmath>
using namespace std;
class Point
{
private:
int x;
int y;
public:
Point(int xx, int yy) : x(xx), y(yy) {}
int getX()
{
return x;
}
int getY()
{
return y;
}
};
class Line
{
private:
float length;
Point p1, p2;
public:
Line(Point a, Point b) : p1(a), p2(b)
{
length = sqrt((p1.getX() - p2.getX()) * (p1.getX() - p2.getX()) + (p1.getY() - p2.getY()) * (p1.getY() - p2.getY()));
}
float getLength()
{
return length;
}
};
int main()
{
Point p1(4, 4), p2(2, 2);
Line L(p1, p2);
cout << L.getLength();
return 0;
}
```
此代码定义了两个类:Point代表一个点,Line代表一条直线。通过构造函数初始化Point类的对象,并在Line类的构造函数中计算并赋值length的值。getLength函数返回length的值。在主函数中创建Point对象和Line对象,并输出计算得到的直线长度。
阅读全文
相关推荐
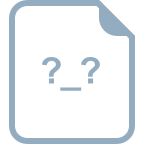
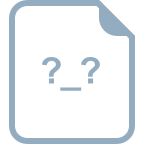














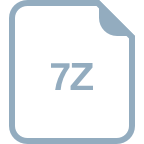
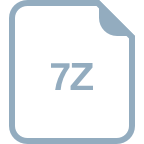