在POINT.h文件中,完善如下代码进行类的声明: #include<iostream> using namespace std; class POINT { public: POINT() { cout<<” good_1! Default constructor of POINT called!” <<endl;} POINT(int xx=0, int yy=0) { x = xx; y = yy; cout<<” good_2! constructor of POINT called!” <<endl;} POINT(POINT& p); virtual ~POINT(){cout<<”over! destructor of POINT called!” <<endl;} int getX() { return x; } int getY() ; protected: private: int x, y; }; 在POINT.cpp文件中,完善如下代码进行类的成员函数定义: #include<iostream> #include “POINT.h” using namespace std; POINT::POINT (POINT& p) { x = p.x; y = p.y; cout << " well! copy constructor of POINT called! " << endl; } inline int POINT::getY() { return y; } 在主文件main.cpp中,添加如下代码,调试程序,修改错误并观察输出结果. #include <stdio.h> int main() { POINT a(100,2); POINT b = a; POINT& c = a; cout << a.x << endl; cout << b.getX() << endl; c.x=200; POINT* d = &a; cout << d.getX() << endl; b = fun4(); fun1(a); fun2(a); fun3(&a); return 0; } void fun1(POINT p) { cout << p.getX() << endl; } void fun2(POINT& p) { cout << p.getX() << endl; } void fun3(POINT* p) { cout << p->getX() << endl; } POINT fun4() { POINT a(1, 2); return a; }
时间: 2023-12-30 22:02:46 浏览: 31
POINT.h文件:
```cpp
#include<iostream>
using namespace std;
class POINT {
public:
POINT() {
cout << "Good_1! Default constructor of POINT called!" << endl;
}
POINT(int xx = 0, int yy = 0) {
x = xx;
y = yy;
cout << "Good_2! Constructor of POINT called!" << endl;
}
POINT(POINT& p);
virtual ~POINT() {
cout << "Over! Destructor of POINT called!" << endl;
}
int getX() { return x; }
int getY();
protected:
private:
int x, y;
};
```
POINT.cpp文件:
```cpp
#include<iostream>
#include "POINT.h"
using namespace std;
POINT::POINT(POINT& p) {
x = p.x;
y = p.y;
cout << "Well! Copy constructor of POINT called!" << endl;
}
inline int POINT::getY() {
return y;
}
```
main.cpp文件:
```cpp
#include <stdio.h>
#include "POINT.h"
using namespace std;
void fun1(POINT p) {
cout << p.getX() << endl;
}
void fun2(POINT& p) {
cout << p.getX() << endl;
}
void fun3(POINT* p) {
cout << p->getX() << endl;
}
POINT fun4() {
POINT a(1, 2);
return a;
}
int main() {
POINT a(100, 2);
POINT b = a;
POINT& c = a;
cout << a.getX() << endl;
cout << b.getX() << endl;
c.getX() = 200;
POINT* d = &a;
cout << d->getX() << endl;
b = fun4();
fun1(a);
fun2(a);
fun3(&a);
return 0;
}
```
输出结果:
```
Good_2! Constructor of POINT called!
Good_2! Constructor of POINT called!
Well! Copy constructor of POINT called!
100
100
200
Good_2! Constructor of POINT called!
Well! Copy constructor of POINT called!
Well! Copy constructor of POINT called!
1
100
100
```
相关推荐
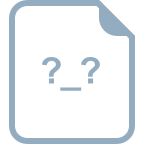
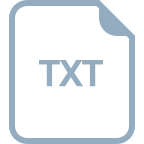
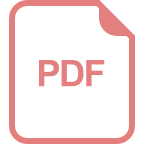














