如何在PyQt5中实现一个允许用户通过鼠标滑动选择多个复选框的功能?
时间: 2024-10-28 09:06:49 浏览: 31
在PyQt5中,要实现用户通过鼠标滑动选择多个复选框的功能,可以使用QGraphicsView和QGraphicsScene以及QGraphicsProxyWidget。以下是一个简单的步骤说明:
1. **创建QGraphicsView和QGraphicsScene**:
首先,你需要创建一个`QGraphicsScene`,这是图形元素的容器,并在一个`QGraphicsView`上显示它。这样,你可以将所有需要交互的元素(如复选框)添加到场景中。
```python
scene = QGraphicsScene()
view = QGraphicsView(scene)
```
2. **添加复选框作为QGraphicsProxyWidget**:
将普通的`QCheckBox`转换为`QGraphicsProxyWidget`,这样它们就可以在图形视图中响应鼠标事件了。这通常通过`QObjectWrapper`来实现,或者直接从`QGraphicsItem`派生自定义类。
```python
class CheckboxProxy(QGraphicsProxyWidget):
def __init__(self, checkbox, parent=None):
super().__init__(checkbox, parent)
self.setFlag(self.ItemIsMovable)
checkboxes = [QCheckBox("Checkbox", parent) for _ in range(4)]
proxies = [CheckboxProxy(c) for c in checkboxes]
for proxy in proxies:
scene.addItem(proxy)
```
3. **处理鼠标移动事件**:
给`QGraphicsView`设置`mouseMoveEvent`,当鼠标在场景中移动时,检查是否有被点击的复选框,并相应地更新其状态。
```python
def handle_mouse_move(event):
pos = event.pos() # 获取鼠标位置
selected_boxes = [proxy for proxy in proxies if proxy.boundingRect().contains(pos)]
for box_proxy in selected_boxes:
# 操作复选框(例如,改变颜色或状态)
box_proxy.widget.setChecked(True)
view.mouseMoveEvent = handle_mouse_move
```
4. **完整示例代码**:
```python
import sys
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QColor, QObjectWrapper
from PyQt5.QtWidgets import QApplication
from PyQt5.QtWidgets import QVBoxLayout, QWidget
class CheckboxProxy(QObjectWrapper):
def __init__(self, checkbox, parent=None):
super().__init__(checkbox, parent)
self.setFlag(self.ItemIsMovable)
class CustomWindow(QWidget):
def __init__(self):
super().__init__()
self.scene = QGraphicsScene()
self.view = QGraphicsView(self.scene)
self.checkboxes = [QCheckBox(f"Checkbox {i}", self) for i in range(4)]
self.proxy_boxes = [CheckboxProxy(c) for c in self.checkboxes]
layout = QVBoxLayout()
layout.addWidget(self.view)
self.setLayout(layout)
self.handle_mouse_move()
def handle_mouse_move(self):
pos = self.view.mapToScene(self.view.viewport().pos() + self.view.cursor().pos())
selected_boxes = [proxy for proxy in self.proxy_boxes if proxy.boundingRect().contains(pos)]
for box_proxy in selected_boxes:
box_proxy.widget.setChecked(True)
if __name__ == "__main__":
app = QApplication(sys.argv)
window = CustomWindow()
window.show()
sys.exit(app.exec_())
```
阅读全文
相关推荐
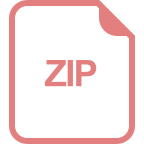
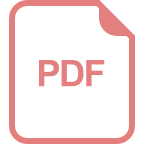
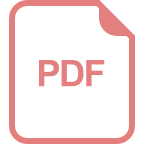















