std::ifstream读取整个文件
时间: 2023-07-08 17:56:16 浏览: 103
您可以使用以下代码来使用 `std::ifstream` 读取整个文件:
```
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("filename.txt");
if (file.is_open()) {
std::string content((std::istreambuf_iterator<char>(file)),
(std::istreambuf_iterator<char>()));
std::cout << content << std::endl;
file.close();
} else {
std::cerr << "Unable to open file" << std::endl;
}
return 0;
}
```
在此代码中,我们使用 `std::ifstream` 打开文件并检查文件是否成功打开。如果文件已成功打开,我们使用 `std::istreambuf_iterator` 从文件中读取所有内容并将其存储在字符串中。最后,我们输出内容并关闭文件。
请注意,这种方法适用于小型文件,对于大型文件,最好使用逐行读取或分块读取等技术。
相关问题
std::ifstream读取文件
您好!对于使用std::ifstream读取文件,您可以按照以下步骤进行操作:
1. 包含头文件:`#include <fstream>`
2. 声明std::ifstream对象并打开文件:`std::ifstream file("filename.txt");`
这里的"filename.txt"是您要读取的文件名。如果文件在与您的源代码相同的目录下,可以直接使用文件名;否则,您需要提供文件的完整路径。
3. 检查文件是否成功打开:可以使用`is_open()`函数来检查文件是否成功打开。
例如:`if (file.is_open()) { /* 文件成功打开 */ } else { /* 打开失败 */ }`
4. 读取文件内容:使用`operator>>`或`getline()`等函数来读取文件的内容。
例如:
```cpp
std::string line;
while (std::getline(file, line)) {
// 处理每一行的内容
}
```
5. 关闭文件:在读取完文件内容后,使用`close()`函数关闭文件。
例如:`file.close();`
请注意,这只是一个简单的示例代码,您可以根据自己的需求进行适当的修改和扩展。同时,还要确保读取的文件路径和文件名正确,并且文件存在且可读。
希望这能对您有所帮助!如果您还有其他问题,请随时提问。
使用 std::ifstream 类读取文件
可以使用 `std::ifstream` 类来读取文件。以下是一个简单的例子:
```c++
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("example.txt");
if (file.is_open()) {
std::string line;
while (getline(file, line)) {
std::cout << line << '\n';
}
file.close();
} else {
std::cout << "Unable to open file\n";
}
return 0;
}
```
在此示例中,我们打开名为 `example.txt` 的文件,并检查它是否成功打开。如果打开成功,我们使用 `getline()` 函数从文件中逐行读取数据,并将其打印到标准输出流中。最后,我们关闭文件。如果打开文件失败,我们打印一条错误消息。
请注意,在使用 `std::ifstream` 读取文件时,需要包含头文件 `<fstream>`。
阅读全文
相关推荐
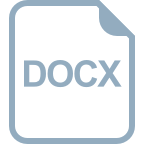
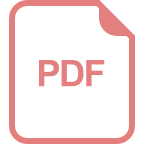
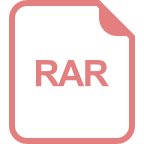













