pso-bp python
时间: 2023-09-08 20:14:40 浏览: 207
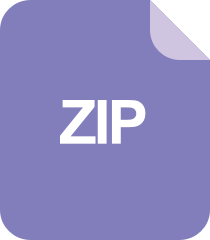
PSO-BP 回归预测 python语言实现

PSO-BP 是一种结合了粒子群优化算法(Particle Swarm Optimization,PSO)和反向传播算法(Back Propagation,BP)的神经网络训练方法,它能够有效地提高神经网络的训练效率和精度。在 Python 中,可以使用 NumPy 库来实现 PSO-BP 算法。
以下是一个简单的 PSO-BP 神经网络训练的 Python 实现示例:
```python
import numpy as np
# 定义神经网络模型
class NeuralNetwork:
def __init__(self, input_dim, hidden_dim, output_dim):
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.output_dim = output_dim
self.w1 = np.random.randn(input_dim, hidden_dim)
self.b1 = np.zeros((1, hidden_dim))
self.w2 = np.random.randn(hidden_dim, output_dim)
self.b2 = np.zeros((1, output_dim))
# 前向传播函数
def forward(self, x):
z1 = np.dot(x, self.w1) + self.b1
a1 = np.tanh(z1)
z2 = np.dot(a1, self.w2) + self.b2
a2 = np.tanh(z2)
return a2
# 计算神经网络损失函数
def loss(self, x, y):
y_pred = self.forward(x)
loss = np.sum((y - y_pred) ** 2) / len(x)
return loss
# 反向传播函数
def backward(self, x, y, learning_rate):
# 前向传播
z1 = np.dot(x, self.w1) + self.b1
a1 = np.tanh(z1)
z2 = np.dot(a1, self.w2) + self.b2
a2 = np.tanh(z2)
# 计算输出层和隐层的误差
delta2 = (y - a2) * (1 - np.tanh(z2) ** 2)
delta1 = np.dot(delta2, self.w2.T) * (1 - np.tanh(z1) ** 2)
# 更新权重和偏置
self.w2 += learning_rate * np.dot(a1.T, delta2)
self.b2 += learning_rate * np.sum(delta2, axis=0, keepdims=True)
self.w1 += learning_rate * np.dot(x.T, delta1)
self.b1 += learning_rate * np.sum(delta1, axis=0, keepdims=True)
# 粒子类
class Particle:
def __init__(self, nn, learning_rate, w1_vel, b1_vel, w2_vel, b2_vel):
self.nn = nn
self.learning_rate = learning_rate
self.w1_vel = w1_vel
self.b1_vel = b1_vel
self.w2_vel = w2_vel
self.b2_vel = b2_vel
# 计算适应度函数
def fitness(self, x, y):
loss = self.nn.loss(x, y)
fitness = 1 / (1 + loss)
return fitness
# 更新粒子的位置和速度
def update(self, global_best_pos, w, c1, c2):
# 更新速度
self.w1_vel = w * self.w1_vel + c1 * np.random.rand(*self.nn.w1.shape) * (self.nn.best_w1 - self.nn.w1) + c2 * np.random.rand(*self.nn.w1.shape) * (global_best_pos[0] - self.nn.w1)
self.b1_vel = w * self.b1_vel + c1 * np.random.rand(*self.nn.b1.shape) * (self.nn.best_b1 - self.nn.b1) + c2 * np.random.rand(*self.nn.b1.shape) * (global_best_pos[1] - self.nn.b1)
self.w2_vel = w * self.w2_vel + c1 * np.random.rand(*self.nn.w2.shape) * (self.nn.best_w2 - self.nn.w2) + c2 * np.random.rand(*self.nn.w2.shape) * (global_best_pos[2] - self.nn.w2)
self.b2_vel = w * self.b2_vel + c1 * np.random.rand(*self.nn.b2.shape) * (self.nn.best_b2 - self.nn.b2) + c2 * np.random.rand(*self.nn.b2.shape) * (global_best_pos[3] - self.nn.b2)
# 更新权重和偏置
self.nn.w1 += self.learning_rate * self.w1_vel
self.nn.b1 += self.learning_rate * self.b1_vel
self.nn.w2 += self.learning_rate * self.w2_vel
self.nn.b2 += self.learning_rate * self.b2_vel
# 粒子群优化算法
class PSO:
def __init__(self, nn, learning_rate, num_particles, num_epochs, w, c1, c2):
self.nn = nn
self.learning_rate = learning_rate
self.num_particles = num_particles
self.num_epochs = num_epochs
self.w = w
self.c1 = c1
self.c2 = c2
self.particles = []
for i in range(num_particles):
particle = Particle(nn, learning_rate, np.zeros(nn.w1.shape), np.zeros(nn.b1.shape), np.zeros(nn.w2.shape), np.zeros(nn.b2.shape))
self.particles.append(particle)
# 训练神经网络
def train(self, x, y):
global_best_fitness = -np.inf
global_best_pos = None
for epoch in range(self.num_epochs):
for i in range(self.num_particles):
particle = self.particles[i]
fitness = particle.fitness(x, y)
if fitness > particle.nn.best_fitness:
particle.nn.best_fitness = fitness
particle.nn.best_w1 = particle.nn.w1
particle.nn.best_b1 = particle.nn.b1
particle.nn.best_w2 = particle.nn.w2
particle.nn.best_b2 = particle.nn.b2
if fitness > global_best_fitness:
global_best_fitness = fitness
global_best_pos = (particle.nn.best_w1, particle.nn.best_b1, particle.nn.best_w2, particle.nn.best_b2)
particle.update(global_best_pos, self.w, self.c1, self.c2)
self.nn.w1 = global_best_pos[0]
self.nn.b1 = global_best_pos[1]
self.nn.w2 = global_best_pos[2]
self.nn.b2 = global_best_pos[3]
```
以上代码实现了一个 PSO-BP 神经网络训练算法。其中,NeuralNetwork 类定义了神经网络模型,Particle 类定义了粒子的属性和行为,PSO 类定义了 PSO 算法的属性和行为。可以使用这个类来训练神经网络并进行预测。
阅读全文
相关推荐
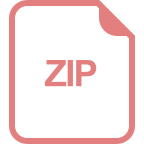
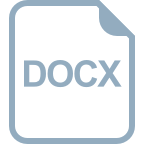













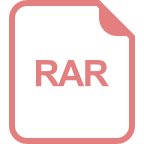