怎么查看等待CPU的进程数
时间: 2023-08-15 13:05:28 浏览: 56
在Linux系统中,可以使用以下命令来查看等待CPU的进程数:
1. top命令:在top命令的交互界面中,按下键盘上的“1”键,可以查看每个CPU核心的使用情况,其中“%CPU”一列下方的“wa”表示等待I/O操作的时间,如果这个值很高,说明有很多进程在等待I/O操作,也就是等待CPU资源。
2. mpstat命令:使用mpstat命令可以查看每个CPU核心的使用率情况,其中“%iowait”列表示等待I/O操作的时间百分比,如果这个值很高,说明有很多进程在等待I/O操作,也就是等待CPU资源。
3. vmstat命令:使用vmstat命令可以查看系统的CPU使用率、内存使用率、磁盘I/O等情况,其中“wa”列表示等待I/O操作的时间百分比,如果这个值很高,说明有很多进程在等待I/O操作,也就是等待CPU资源。
4. sar命令:使用sar命令可以查看系统的历史负载情况,包括CPU使用率、内存使用率、磁盘I/O等情况,其中“%iowait”列表示等待I/O操作的时间百分比,如果这个值很高,说明有很多进程在等待I/O操作,也就是等待CPU资源。
通过以上命令,可以查看等待CPU的进程数和时间百分比等情况,从而评估系统的性能和健康状态。
相关问题
linux top 查看cpu详解
Linux系统的top命令是一个非常实用的工具,用来实时地监控系统的状态,包括CPU、内存、磁盘等方面。在top命令中,我们可以通过按下键盘上的不同字母键来切换显示不同的信息,下面我主要讲解一下如何使用top命令查看CPU的使用情况。
1. 打开终端,输入top命令,进入top命令的交互界面。
2. 在交互界面中,可以看到很多信息,其中第一行显示了系统的总体情况,包括系统当前时间、运行时间、登录用户数、负载平均值等。CPU使用情况则显示在第三行。
3. 第三行中,分别显示了CPU的总体使用情况和各个进程的CPU使用情况。其中,%Cpu(s)表示CPU的总体使用情况,us表示用户态占用CPU的百分比,sy表示内核态占用CPU的百分比,ni表示优先级较低的进程占用CPU的百分比,id表示CPU空闲的百分比,wa表示等待IO操作的进程占用CPU的百分比,hi表示硬件中断的占用CPU的百分比,si表示软件中断的占用CPU的百分比。在八个百分比的后面,分别显示了CPU的逻辑核心数。
4. 除了第三行的信息,我们还可以按下键盘上的1键,来查看每个CPU逻辑核心的使用情况。在这个界面中,我们可以看到每个逻辑核心的使用情况,包括用户态、内核态、空闲态和等待IO操作的进程等。
5. 除了以上的方式,我们还可以按下键盘上的t键来切换显示不同的信息,包括进程的状态、内存的使用情况等。
通过使用top命令,我们可以实时地监控系统的CPU使用情况,及时发现系统中的CPU瓶颈,有助于我们及时调整系统的配置,保证系统的稳定性和性能。
cpu load计算方式
CPU Load是指系统中正在运行或等待运行的进程数量。在Linux系统中,CPU Load的计算方式如下:
CPU Load = (正在运行的进程数 + 等待CPU资源的进程数) / CPU核心数
其中,正在运行的进程数指的是当前正在执行的进程数,等待CPU资源的进程数指的是正在等待CPU时间片的进程数。CPU核心数指的是系统中的CPU核心数,可以通过命令“cat /proc/cpuinfo | grep processor | wc -l”来查看。
例如,假设系统中有4个CPU核心,正在运行的进程数为2个,等待CPU资源的进程数为1个,则CPU Load的计算如下:
CPU Load = (2 + 1) / 4 = 0.75
因此,当前系统的CPU Load为0.75。当CPU Load超过1时,意味着系统中有更多的进程在等待CPU资源,此时系统的响应速度可能会变慢。因此,在评估系统性能时,需要时刻关注CPU Load的情况。
相关推荐
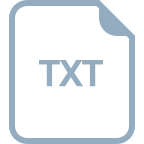
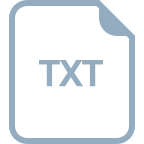
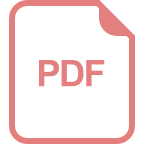












