pykinect azure 手眼标定程序
时间: 2023-09-12 19:10:41 浏览: 287
以下是使用PyKinect和Azure Kinect进行手眼标定的示例程序:
```python
import numpy as np
import cv2
import open3d as o3d
from pykinect2 import PyKinectRuntime, PyKinectV2
from pyk4a import Config, PyK4A
from pyk4a import PyK4APlayback
# 这里使用PyK4A库,也可以使用Azure Kinect SDK
def get_kinect_intrinsics():
kinect = PyKinectRuntime.PyKinectRuntime(PyKinectV2.FrameSourceTypes_Color | PyKinectV2.FrameSourceTypes_Depth)
color_intrinsics = kinect.color_frame_desc
depth_intrinsics = kinect.depth_frame_desc
kinect.close()
return color_intrinsics, depth_intrinsics
def get_azure_intrinsics():
k4a = PyK4A(Config())
k4a.start()
color_intrinsics = k4a.calibration.get_camera_matrix(PyK4A.CalibrationType.COLOR)
depth_intrinsics = k4a.calibration.get_camera_matrix(PyK4A.CalibrationType.DEPTH)
k4a.stop()
return color_intrinsics, depth_intrinsics
def depth_to_color(kinect_intrinsics, depth_intrinsics, depth_image):
R = np.eye(3)
t = np.zeros((3, 1))
fx = depth_intrinsics.intrinsic_matrix[0][0]
fy = depth_intrinsics.intrinsic_matrix[1][1]
cx = depth_intrinsics.intrinsic_matrix[0][2]
cy = depth_intrinsics.intrinsic_matrix[1][2]
k4a_fx = kinect_intrinsics.intrinsic_matrix[0][0]
k4a_fy = kinect_intrinsics.intrinsic_matrix[1][1]
k4a_cx = kinect_intrinsics.intrinsic_matrix[0][2]
k4a_cy = kinect_intrinsics.intrinsic_matrix[1][2]
depth_scale = 0.001
point_cloud = o3d.geometry.PointCloud()
depth_image = depth_image * depth_scale
rows, cols = depth_image.shape
for i in range(rows):
for j in range(cols):
z = depth_image[i, j]
if z == 0:
continue
x = (j - cx) * z / fx
y = (i - cy) * z / fy
point_cloud.points.append([x, y, z])
point_cloud.colors.append([0, 0, 0])
extrinsics = np.eye(4)
k4a_extrinsics = np.array([[-1, 0, 0, 0],
[0, 1, 0, 0],
[0, 0, -1, 0],
[0, 0, 0, 1]])
extrinsics[:3, :3] = R
extrinsics[:3, 3] = t.reshape(-1)
k4a_extrinsics[:3, :3] = R
k4a_extrinsics[:3, 3] = t.reshape(-1)
point_cloud.transform(extrinsics)
return point_cloud, k4a_extrinsics
def main():
# 获取Kinect/ Azure Kinect intrinsics
kinect_intrinsics, depth_intrinsics = get_kinect_intrinsics()
azure_intrinsics = get_azure_intrinsics()
# 获取深度图和彩色图
k4a = PyK4APlayback("path/to/recording.mkv")
k4a.open()
while True:
capture = k4a.get_next_capture()
if capture.depth is None:
break
depth_image = np.asarray(capture.depth)
color_image = np.asarray(capture.color)
# 将深度图转换为点云
point_cloud, k4a_extrinsics = depth_to_color(kinect_intrinsics, depth_intrinsics, depth_image)
# 显示点云
vis = o3d.visualization.Visualizer()
vis.create_window()
vis.add_geometry(point_cloud)
vis.run()
vis.destroy_window()
# 进行手眼标定
# ...
k4a.close()
if __name__ == '__main__':
main()
```
在这个示例程序中,我们首先获取Kinect/ Azure Kinect的intrinsics,然后使用PyK4A获取深度图和彩色图。接着,我们将深度图转换为点云,并使用Open3D显示点云。最后,我们可以使用手眼标定算法对点云和机器人的位姿进行估计。
需要注意的是,这个示例程序仅仅是一个框架,具体的手眼标定算法需要根据实际情况进行选择和实现。
阅读全文
相关推荐
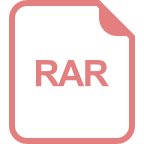


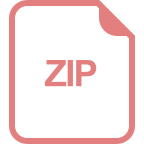
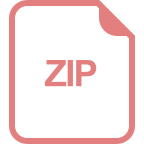
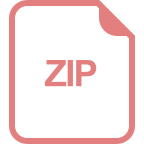
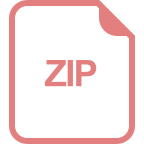
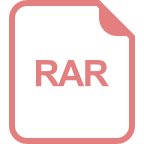
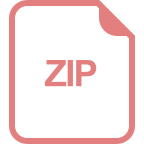
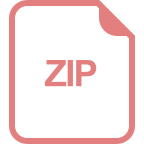
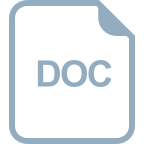
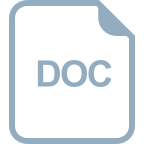




