可以帮我编写一个基于python使用pykinect2调用kinect,同时获取深度图像与彩色图像,每隔30秒保存为时间名字的代码吗
时间: 2023-06-06 14:08:55 浏览: 58
可以的,以下是基于python使用pykinect2调用kinect获取深度图像与彩色图像并保存的代码示例:
```python
import ctypes
import _ctypes
import pygame
import sys
import numpy as np
from pykinect2 import PyKinectV2
from pykinect2.PyKinectV2 import *
class Kinect(object):
def __init__(self):
pygame.init()
self.kinect = PyKinectRuntime.PyKinectRuntime(PyKinectV2.FrameSourceTypes_Color | PyKinectV2.FrameSourceTypes_Depth)
self.depth_width, self.depth_height = self.kinect.depth_frame_desc.Width, self.kinect.depth_frame_desc.Height
self.color_width, self.color_height = self.kinect.color_frame_desc.Width, self.kinect.color_frame_desc.Height
self.depth_array = np.zeros((self.depth_height, self.depth_width), dtype=np.uint16)
self.color_array = np.zeros((self.color_height, self.color_width, 4), dtype=np.uint8)
self.depth_surface = pygame.Surface((self.depth_width, self.depth_height), 0, 16)
self.color_surface = pygame.Surface((self.color_width, self.color_height), 0, 32)
self.clock = pygame.time.Clock()
def get_depth(self):
if self.kinect.has_new_depth_frame():
frame = self.kinect.get_last_depth_frame()
frame = frame.reshape(self.depth_height, self.depth_width)
np.copyto(self.depth_array, frame)
self.depth_surface = pygame.surfarray.make_surface(self.depth_array)
self.depth_surface = pygame.transform.scale(self.depth_surface, (640, 480))
def get_color(self):
if self.kinect.has_new_color_frame():
frame = self.kinect.get_last_color_frame()
frame = frame.reshape((self.color_height, self.color_width, 4))
np.copyto(self.color_array, frame)
self.color_surface = pygame.surfarray.make_surface(self.color_array)
self.color_surface = pygame.transform.scale(self.color_surface, (640, 480))
def save_frames(self):
depth_filename = str(pygame.time.get_ticks()) + '_depth.png'
color_filename = str(pygame.time.get_ticks()) + '_color.png'
pygame.image.save(self.depth_surface, depth_filename)
pygame.image.save(self.color_surface, color_filename)
def run(self):
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
self.kinect.close()
pygame.quit()
sys.exit()
self.get_depth()
self.get_color()
self.save_frames()
self.clock.tick(30)
pygame.display.update()
if __name__ == '__main__':
kinect = Kinect()
kinect.run()
```
在这段代码中,我们通过`PyKinectRuntime`模块创建了`PyKinectV2`运行时实例,并指定了要获取的帧源类型(彩色和深度)。然后,我们通过`numpy`库创建了对应的图像数组,使用`pygame`库的`Surface`类创建了彩色和深度图像的表面。我们循环读取深度和彩色帧,将它们复制到相应的数组中,然后将图像表面转换为屏幕表面并进行缩放。最后,我们使用`pygame`的`image.save()`方法将彩色和深度图像保存到硬盘上,并通过`clock.tick()`方法控制帧率。
你只需要将这段代码保存到一个名为kinect.py的文件中,并在Python环境中运行它即可。注意:要运行该代码,需要安装 `pykinect2`、`pygame` 以及适用于Windows SDK的Kinect 2.0驱动程序。
相关推荐
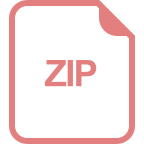
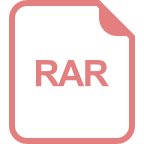














