用php创建一个用于填写用户资料的表单,要求表单内包含文本框、单选按钮、复选框、下拉式列表框及文本区域,并在提交表单后通过表格显示所提交的信息。
时间: 2023-12-30 15:07:07 浏览: 23
以下是一个示例代码,您可以在此基础上自行修改和完善:
```
<!DOCTYPE html>
<html>
<head>
<title>User Profile Form</title>
</head>
<body>
<h1>User Profile Form</h1>
<form action="submit.php" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required><br><br>
<label for="gender">Gender:</label>
<input type="radio" id="male" name="gender" value="male" required>
<label for="male">Male</label>
<input type="radio" id="female" name="gender" value="female">
<label for="female">Female</label><br><br>
<label for="interests">Interests:</label>
<input type="checkbox" id="music" name="interests[]" value="music">
<label for="music">Music</label>
<input type="checkbox" id="sports" name="interests[]" value="sports">
<label for="sports">Sports</label>
<input type="checkbox" id="travel" name="interests[]" value="travel">
<label for="travel">Travel</label><br><br>
<label for="country">Country:</label>
<select id="country" name="country">
<option value="USA">USA</option>
<option value="China">China</option>
<option value="Japan">Japan</option>
<option value="Korea">Korea</option>
</select><br><br>
<label for="message">Message:</label>
<textarea id="message" name="message" rows="5" cols="30"></textarea><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
```
这个表单包含了文本框、单选按钮、复选框、下拉式列表框以及文本区域。其中,文本框和单选按钮都是必填项,而复选框、下拉式列表框和文本区域是可选项。在提交表单后,表单数据将被传递到 `submit.php` 文件进行处理。下面是 `submit.php` 文件的示例代码:
```
<!DOCTYPE html>
<html>
<head>
<title>User Profile</title>
</head>
<body>
<h1>User Profile</h1>
<table>
<tr>
<th>Name:</th>
<td><?php echo $_POST["name"]; ?></td>
</tr>
<tr>
<th>Gender:</th>
<td><?php echo $_POST["gender"]; ?></td>
</tr>
<tr>
<th>Interests:</th>
<td><?php echo implode(", ", $_POST["interests"]); ?></td>
</tr>
<tr>
<th>Country:</th>
<td><?php echo $_POST["country"]; ?></td>
</tr>
<tr>
<th>Message:</th>
<td><?php echo $_POST["message"]; ?></td>
</tr>
</table>
</body>
</html>
```
在这个文件中,我们使用了 PHP 的 `$_POST` 变量来获取表单数据,并将这些数据显示在一个表格中。需要注意的是,我们使用了 `implode` 函数来将复选框的值合并为一个字符串。
相关推荐
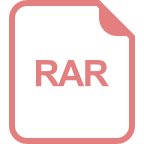
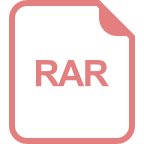
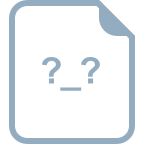
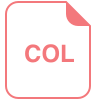
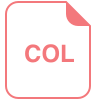












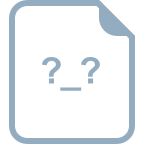