c++ string字符串删除空格
时间: 2023-07-21 13:06:24 浏览: 186
可以使用C++标准库的`erase`和`remove_if`函数来删除字符串中的空格。
具体实现代码如下:
```c++
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
int main()
{
string str = " hello world! ";
str.erase(remove_if(str.begin(), str.end(), ::isspace), str.end());
cout << str << endl;
return 0;
}
```
上述代码中,`remove_if`函数接受一个用于判断是否为需要删除元素的谓词函数,这里使用了`::isspace`函数,它能够判断一个字符是否为空格字符。`erase`函数则用于删除满足条件的元素。
相关问题
c++去掉字符串首尾空格
在 C++ 中,你可以使用标准库提供的 `std::trim` 函数或者简单的循环来去除字符串首尾的空格。这里提供两种常见的方法:
1. 使用 `std::string` 的 `erase` 和 `find_first_not_of` 方法:
```cpp
#include <algorithm>
#include <cctype>
std::string trimString(std::string str) {
str.erase(0, str.find_first_not_of(' '));
str.erase(str.find_last_not_of(' ') + 1);
return str;
}
```
2. 使用 C 语言风格的迭代器和 `std::remove_if`:
```cpp
#include <iterator>
#include <algorithm>
std::string trimString(std::string str) {
str.erase(remove_if(str.begin(), str.end(), isspace), str.end());
return str;
}
// 注意你需要包含 `<cctype>` 包导入 isspace 函数
```
这两种方法都会返回一个新的字符串,原始字符串不会被修改。
c++ string空格分割字符串
可以使用 stringstream 和 getline 函数来实现空格分割字符串,示例代码如下:
```c++
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using namespace std;
int main() {
string s = "hello world";
stringstream ss(s);
vector<string> tokens;
string token;
while (getline(ss, token, ' ')) {
tokens.push_back(token);
}
for (const auto& t : tokens) {
cout << t << endl;
}
return 0;
}
```
输出结果为:
```
hello
world
```
阅读全文
相关推荐
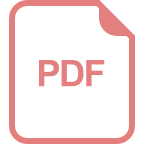













