python fastapi 使用rabbitmq
时间: 2024-06-05 17:04:09 浏览: 418
Python FastAPI 是一个快速(高性能)且易于学习的 Web 框架,它可以与多种消息队列(MQ)进行集成,其中包括 RabbitMQ。
在使用 FastAPI 和 RabbitMQ 进行集成时,你可以使用 Pika 库来连接 RabbitMQ,然后在 FastAPI 中使用 FastAPI-BackgroundTasks 库来异步处理消息队列中的消息。以下是一个简单的示例:
1. 安装 FastAPI 和 Pika 库:
```python
pip install fastapi
pip install pika
pip install fastapi-background-tasks
```
2. 在 FastAPI 应用程序中导入相关库:
```python
from fastapi import FastAPI, BackgroundTasks
import pika
```
3. 连接 RabbitMQ:
```python
connection = pika.BlockingConnection(pika.ConnectionParameters(host='localhost'))
channel = connection.channel()
channel.queue_declare(queue='my_queue')
```
4. 编写处理函数,并在函数中使用 `channel.basic_publish()` 方法将消息发布到消息队列中:
```python
def process_message(message):
# 处理消息的代码
print("Received message:", message)
def send_message_to_queue(message):
channel.basic_publish(exchange='', routing_key='my_queue', body=message)
```
5. 在 FastAPI 中定义异步任务:
```python
app = FastAPI()
@app.post("/send_message")
async def send_message(message: str, background_tasks: BackgroundTasks):
background_tasks.add_task(send_message_to_queue, message)
return {"message": "Message sent successfully!"}
@app.on_event("startup")
async def startup_event():
connection = pika.BlockingConnection(pika.ConnectionParameters(host='localhost'))
channel = connection.channel()
channel.queue_declare(queue='my_queue')
channel.basic_consume(queue='my_queue', on_message_callback=process_message, auto_ack=True)
channel.start_consuming()
```
6. 启动应用程序:
```python
uvicorn main:app --reload
```
这是一个简单的示例,你可以根据你的需求进行调整和扩展。下面是一些相关问题:
阅读全文
相关推荐
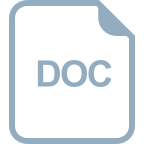
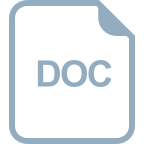
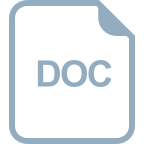

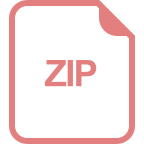

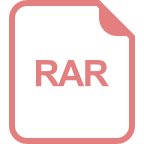
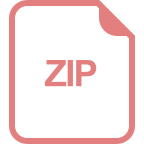
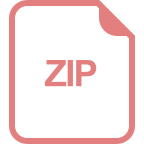
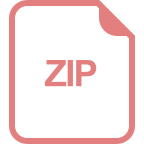
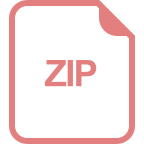
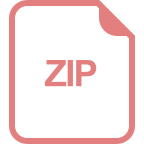
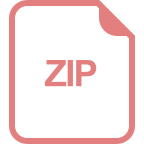
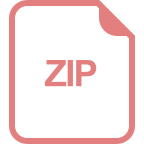
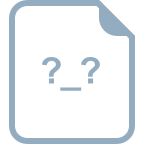
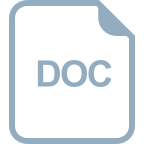
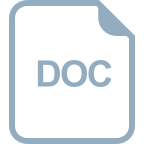
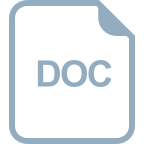
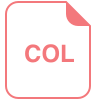