opencv+yolov5+c++
时间: 2023-09-12 11:10:11 浏览: 170
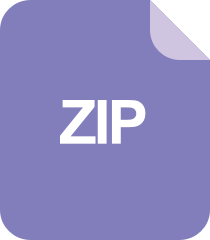
基于Python+Yolov5车牌检测,更快更准
OpenCV是一个开源的计算机视觉库,用于图像和视频处理。YOLOv5是一种目标检测算法,可以在图像中识别出物体的位置和类别。C++是一种编程语言,可以用于实现图像处理和机器学习算法。
如果你想使用OpenCV和YOLOv5实现目标检测,可以使用C++编写代码。首先,需要安装OpenCV和YOLOv5,并且配置好相关的环境变量。
然后,可以使用OpenCV读取图像或者视频,并且使用YOLOv5进行目标检测。具体的步骤包括:
1. 加载YOLOv5模型
2. 读取图像或者视频
3. 对于每一帧图像,使用YOLOv5进行目标检测
4. 在图像上绘制检测结果
5. 显示处理后的图像或者视频
这里是一个示例代码,可以用于实现基于OpenCV和YOLOv5的目标检测:
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace std;
using namespace cv;
int main()
{
// 加载YOLOv5模型
string model_path = "yolov5s.pt";
cv::dnn::Net net = cv::dnn::readNet(model_path, "", "ONNX");
// 打开摄像头
VideoCapture cap(0);
if (!cap.isOpened()) {
cerr << "Error opening camera." << endl;
return -1;
}
while (true) {
Mat frame;
cap >> frame;
// 对于每一帧图像,使用YOLOv5进行目标检测
cv::dnn::Blob inputBlob = cv::dnn::blobFromImage(frame, 1 / 255.0, cv::Size(640, 640), cv::Scalar(0, 0, 0), true, false);
net.setInput(inputBlob);
vector<Mat> outs;
net.forward(outs, net.getUnconnectedOutLayersNames());
// 绘制检测结果
float confThreshold = 0.5;
for (size_t i = 0; i < outs.size(); ++i) {
// 获取输出层的信息
Mat detectionMat = outs[i];
for (int j = 0; j < detectionMat.rows; ++j) {
const int probability_index = 5;
const int probability_size = detectionMat.cols - probability_index;
float *prob_array_ptr = &detectionMat.at<float>(j, probability_index);
// 获取最大概率的类别
int objectClass = std::max_element(prob_array_ptr, prob_array_ptr + probability_size) - prob_array_ptr;
// 获取检测框的位置和大小
float confidence = detectionMat.at<float>(j, objectClass + probability_index);
if (confidence > confThreshold) {
int centerX = (int)(detectionMat.at<float>(j, 0) * frame.cols);
int centerY = (int)(detectionMat.at<float>(j, 1) * frame.rows);
int width = (int)(detectionMat.at<float>(j, 2) * frame.cols);
int height = (int)(detectionMat.at<float>(j, 3) * frame.rows);
int left = centerX - width / 2;
int top = centerY - height / 2;
// 绘制检测框和类别
rectangle(frame, Point(left, top), Point(left + width, top + height), Scalar(0, 255, 0), 2);
string label = format("%d:%.2f", objectClass, confidence);
int baseLine;
Size labelSize = getTextSize(label, FONT_HERSHEY_SIMPLEX, 0.5, 1, &baseLine);
top = max(top, labelSize.height);
rectangle(frame, Point(left, top - labelSize.height), Point(left + labelSize.width, top + baseLine), Scalar(255, 255, 255), FILLED);
putText(frame, label, Point(left, top), FONT_HERSHEY_SIMPLEX, 0.5, Scalar(0, 0, 0));
}
}
}
// 显示处理后的图像
imshow("Object Detection", frame);
// 按下ESC键退出程序
if (waitKey(1) == 27) break;
}
return 0;
}
```
在这个示例代码中,使用了OpenCV和YOLOv5实现了目标检测,并且可以实时显示处理后的图像。你可以根据自己的需求修改代码,例如修改模型路径、调整检测阈值等。
阅读全文
相关推荐
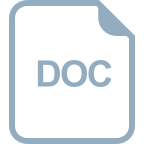
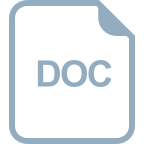

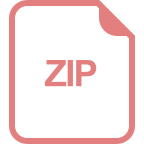
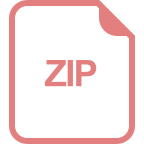
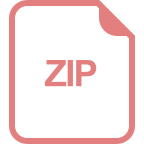
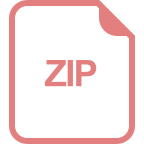
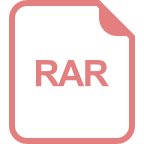
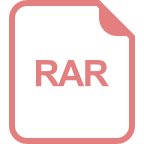
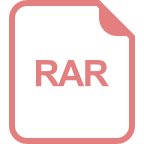
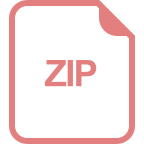
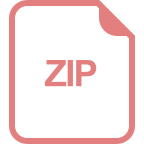
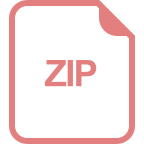
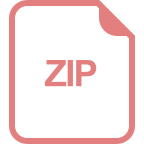


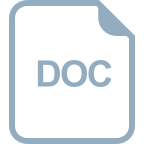
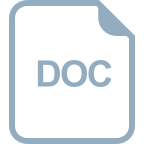