帮我写一段爬取指定网站内容并把内容按照指定编码保存到txt文档并设置指定网页内容为文档名的python程序
时间: 2023-08-31 19:31:23 浏览: 35
### 回答1:
import requests
import osurl = input('请输入网站URL:')response = requests.get(url)
# 获取网页内容
html = response.content
# 获取文件名
filename = os.path.basename(url)
# 设置编码格式
encoding = 'utf-8'
# 写入文件
with open(filename + '.txt', 'w', encoding=encoding) as f:
f.write(html)
### 回答2:
下面是一个示例的Python程序,用于爬取指定网站内容并按照指定编码保存到txt文档,并以指定网页内容作为文档名:
```python
import requests
def crawl_and_save(url, encoding):
# 发送GET请求获取网页内容
response = requests.get(url)
response.encoding = encoding
# 提取网页内容
content = response.text
# 从url中提取文档名
doc_name = url.split("/")[-1]
# 保存到txt文档
with open(f"{doc_name}.txt", "w", encoding=encoding) as file:
file.write(content)
if __name__ == "__main__":
# 指定需要爬取的网站url和编码
url = "https://example.com"
encoding = "utf-8"
# 调用函数进行爬取和保存
crawl_and_save(url, encoding)
```
上述程序通过使用`requests`模块发送GET请求来获取指定网站的内容。然后,使用指定的编码将内容保存到`txt`文档,并使用指定网页内容作为文档名。你可以根据自己的需求修改url和编码的值,将程序适配到你需要爬取的网站中。
### 回答3:
以下是一个简单的Python程序,用于爬取指定网站的内容并将内容按照指定编码保存为txt文档,文档名为指定网页的内容:
```python
import requests
def save_to_txt(url, encoding):
try:
# 发送HTTP请求获取网页内容
response = requests.get(url)
# 设置响应编码
response.encoding = encoding
content = response.text
# 获取指定网页内容作为文档名
doc_name = url.split("/")[-1] + ".txt"
# 将内容保存到txt文档
with open(doc_name, "w", encoding=encoding) as file:
file.write(content)
print("爬取并保存成功!")
except Exception as e:
print("爬取并保存失败!", e)
# 指定要爬取的网页链接
url = "https://example.com"
# 指定编码格式
encoding = "utf-8"
# 调用函数进行爬取和保存
save_to_txt(url, encoding)
```
请注意,上述代码仅用于爬取HTML文本内容,并不包括处理JavaScript渲染的情况。如果网页中包含动态内容,则需要使用更复杂的爬虫技术来获取完整的网页内容。
相关推荐
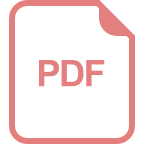
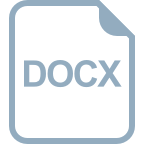
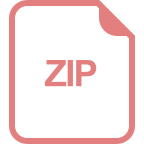













