写一个dht11显示在lcd屏上,并用HCsr04测距的程序,C51
时间: 2023-06-27 09:04:36 浏览: 45
以下是基于C51单片机的DHT11和HC-SR04程序:
```c
#include <reg52.h>
#include <intrins.h>
#define LCD_RS P2_0 //LCD命令/数据选择
#define LCD_RW P2_1 //LCD读/写选择
#define LCD_EN P2_2 //LCD使能信号
#define LCD_DAT P0 //LCD数据口
sbit DHT_DAT = P1^0; //DHT11数据口
sbit TRIG = P1^1; //HC-SR04触发信号
sbit ECHO = P1^2; //HC-SR04回波信号
unsigned char humi, temp; // DHT11湿度和温度
unsigned int distance; // HC-SR04距离
void delay_us(unsigned int us)
{
while(us--)
{
_nop_();
_nop_();
}
}
void delay_ms(unsigned int ms)
{
while(ms--)
{
delay_us(1000);
}
}
void lcd_init()
{
LCD_RS = 0;
LCD_RW = 0;
LCD_EN = 0;
delay_ms(15);
LCD_DAT = 0x30;
LCD_EN = 1;
delay_ms(5);
LCD_EN = 0;
delay_ms(5);
LCD_DAT = 0x30;
LCD_EN = 1;
delay_ms(5);
LCD_EN = 0;
delay_ms(5);
LCD_DAT = 0x30;
LCD_EN = 1;
delay_ms(5);
LCD_EN = 0;
delay_ms(5);
LCD_DAT = 0x38;
LCD_EN = 1;
delay_ms(5);
LCD_EN = 0;
delay_ms(5);
LCD_DAT = 0x08;
LCD_EN = 1;
delay_ms(5);
LCD_EN = 0;
delay_ms(5);
LCD_DAT = 0x01;
LCD_EN = 1;
delay_ms(5);
LCD_EN = 0;
delay_ms(5);
LCD_DAT = 0x06;
LCD_EN = 1;
delay_ms(5);
LCD_EN = 0;
delay_ms(5);
LCD_DAT = 0x0c;
LCD_EN = 1;
delay_ms(5);
LCD_EN = 0;
delay_ms(5);
}
void lcd_write_cmd(unsigned char cmd)
{
LCD_RS = 0;
LCD_RW = 0;
LCD_DAT = cmd;
LCD_EN = 1;
delay_us(5);
LCD_EN = 0;
delay_us(5);
}
void lcd_write_data(unsigned char dat)
{
LCD_RS = 1;
LCD_RW = 0;
LCD_DAT = dat;
LCD_EN = 1;
delay_us(5);
LCD_EN = 0;
delay_us(5);
}
void lcd_clear()
{
lcd_write_cmd(0x01);
delay_ms(5);
}
void lcd_setpos(unsigned char x, unsigned char y)
{
unsigned char pos;
if(x == 0)
{
pos = 0x00 + y;
}
else
{
pos = 0x40 + y;
}
lcd_write_cmd(pos | 0x80);
delay_us(5);
}
void lcd_write_str(unsigned char x, unsigned char y, unsigned char *str)
{
lcd_setpos(x, y);
while(*str)
{
lcd_write_data(*str++);
}
}
void dht11_read()
{
unsigned char i, j;
unsigned char buffer[5] = {0};
DHT_DAT = 0;
delay_ms(20);
DHT_DAT = 1;
delay_us(30);
if(!DHT_DAT)
{
while(!DHT_DAT);
while(DHT_DAT);
for(i=0; i<5; i++)
{
for(j=0; j<8; j++)
{
while(!DHT_DAT);
delay_us(30);
if(DHT_DAT)
{
buffer[i] |= 1 << (7-j);
}
while(DHT_DAT);
}
}
humi = buffer[0];
temp = buffer[2];
}
}
void hc_sr04_read()
{
unsigned int time;
TRIG = 0;
delay_us(2);
TRIG = 1;
delay_us(10);
TRIG = 0;
while(!ECHO);
while(ECHO)
{
time++;
delay_us(1);
}
distance = time / 58;
}
void main()
{
lcd_init();
lcd_clear();
while(1)
{
dht11_read();
hc_sr04_read();
lcd_write_cmd(0x80);
lcd_write_str(0, 0, "Humi:");
lcd_write_data(humi);
lcd_write_str(0, 6, "%");
lcd_write_str(1, 0, "Temp:");
lcd_write_data(temp);
lcd_write_str(1, 6, "C");
lcd_write_cmd(0xc0);
lcd_write_str(2, 0, "Dist:");
lcd_write_data(distance / 100);
lcd_write_data(distance % 100 / 10);
lcd_write_data(distance % 10);
lcd_write_str(2, 3, "cm");
delay_ms(1000);
}
}
```
上述程序中,我们使用DHT11读取环境温度和湿度,使用HC-SR04测量距离,并将它们显示在LCD屏幕上。
相关推荐
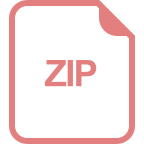
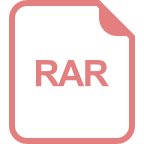














