设计一个金币事件,就比如金币减少怎么设计
时间: 2024-03-11 11:50:32 浏览: 101
假设我们正在开发一个游戏,需要设计一个金币事件,当玩家进行某些操作时,金币数量会发生变化。
首先,需要定义一个金币类 Coin,该类包含以下属性和方法:
```javascript
class Coin {
constructor(amount) {
this.amount = amount; // 金币数量
}
// 增加金币数量
add(amount) {
this.amount += amount;
}
// 减少金币数量
reduce(amount) {
this.amount -= amount;
}
// 获取金币数量
getAmount() {
return this.amount;
}
}
```
接下来,需要设计一个金币事件类 CoinEvent,该类包含以下属性和方法:
```javascript
class CoinEvent {
constructor(coin, amount) {
this.coin = coin; // 金币对象
this.amount = amount; // 金币数量
}
// 执行金币事件
execute() {
// 判断金币数量是否足够
if (this.coin.getAmount() >= this.amount) {
this.coin.reduce(this.amount); // 减少金币数量
console.log(`金币减少了 ${this.amount},当前剩余 ${this.coin.getAmount()} 个金币`);
} else {
console.log(`金币数量不足,当前剩余 ${this.coin.getAmount()} 个金币`);
}
}
}
```
最后,当玩家进行某些操作时,可以创建一个 CoinEvent 对象,然后调用其 execute 方法来执行金币事件。比如:
```javascript
const coin = new Coin(100); // 初始化金币数量为 100
const coinEvent = new CoinEvent(coin, 20); // 创建一个金币事件,减少 20 个金币
coinEvent.execute(); // 执行金币事件
```
以上是一个简单的金币事件的设计和实现,可以根据具体需求进行修改和扩展。
阅读全文
相关推荐
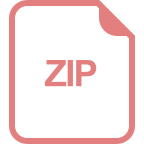
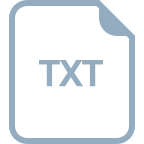
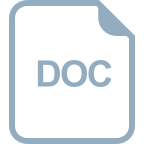
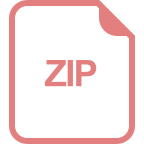
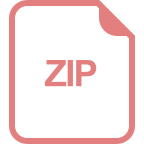
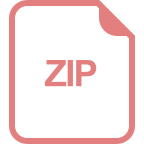
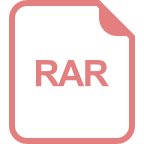
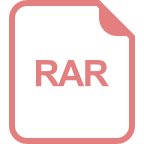
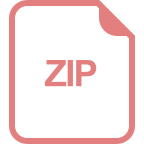
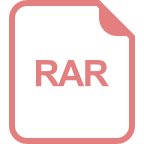
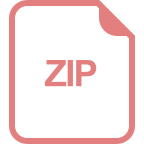
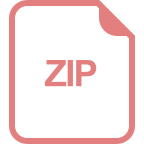
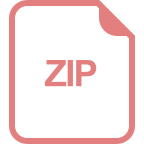
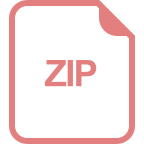
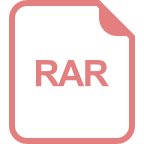
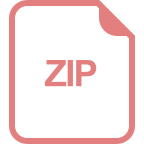
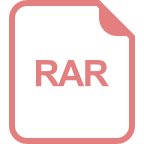
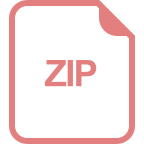
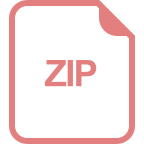