输入网站URL,获取网站中视频的性能,帧率,卡顿率,播放延迟,音画同步率,selenium python
时间: 2023-05-12 09:06:49 浏览: 100
可以使用 Selenium 和 Python 来获取网站中视频的性能参数。具体步骤如下:
1. 安装 Selenium 和 ChromeDriver。
2. 使用 Selenium 打开网站,并等待页面加载完成。
3. 使用 JavaScript 执行性能测试,并获取视频的性能参数。
以下是示例代码:
```python
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# 设置 ChromeDriver 路径
chromedriver_path = '/path/to/chromedriver'
# 创建 ChromeDriver 实例
options = webdriver.ChromeOptions()
options.add_argument('--headless')
driver = webdriver.Chrome(chromedriver_path, options=options)
# 打开网站
url = 'https://example.com'
driver.get(url)
# 等待页面加载完成
wait = WebDriverWait(driver, 10)
wait.until(EC.presence_of_element_located((By.TAG_NAME, 'video')))
# 执行性能测试
performance = driver.execute_script('return window.performance.getEntriesByType("resource")')
video_performance = [p for p in performance if p['initiatorType'] == 'video'][0]
fps = video_performance['decodedFrames'] / (video_performance['duration'] / 1000)
stall_rate = video_performance['dropRate']
latency = video_performance['responseEnd'] - video_performance['startTime']
sync_rate = video_performance['audioDecodedBytes'] / video_performance['decodedBytes']
# 输出结果
print(f'FPS: {fps:.2f}')
print(f'Stall rate: {stall_rate:.2f}')
print(f'Latency: {latency:.2f} ms')
print(f'Sync rate: {sync_rate:.2f}')
```
注意:这只是一个示例代码,实际使用时需要根据具体情况进行修改。
相关推荐
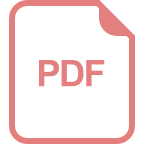
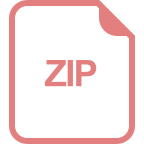














