python爬取贝壳网房屋出售信息,分类存储到本地数据库中(建议sqlite)。 要求:项目源代码
时间: 2024-03-04 20:48:55 浏览: 71
以下是一个简单的 Python 爬虫,用于从贝壳网爬取房屋出售信息,并将其分类存储到本地 SQLite 数据库中。请注意,这只是一个示例,您需要根据您的需求进行修改和改进。同时,爬取网站的行为需要遵守相关法律法规和网站的使用条款。
```python
import requests
from bs4 import BeautifulSoup
import sqlite3
# 定义数据库连接和游标
conn = sqlite3.connect('house.db')
cursor = conn.cursor()
# 创建数据表
cursor.execute('''CREATE TABLE IF NOT EXISTS house
(id INTEGER PRIMARY KEY AUTOINCREMENT,
title TEXT,
location TEXT,
area TEXT,
price TEXT)''')
# 爬取的页面数量
page_num = 5
for page in range(1, page_num + 1):
# 发送 HTTP GET 请求
url = f"https://sz.ke.com/ershoufang/pg{page}/"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 解析 HTML
house_list = soup.find_all('div', {'class': 'info clear'})
# 将数据存储到数据库中
for house in house_list:
title = house.find('div', {'class': 'title'}).text.strip()
location = house.find('div', {'class': 'address'}).text.strip()
area = house.find('div', {'class': 'houseInfo'}).text.strip()
price = house.find('div', {'class': 'totalPrice'}).text.strip()
# 执行 SQL 插入语句
cursor.execute("INSERT INTO house (title, location, area, price) VALUES (?, ?, ?, ?)",
(title, location, area, price))
conn.commit()
# 关闭数据库连接
conn.close()
```
在运行此代码之前,请确保已安装必要的库:requests,beautifulsoup4 和 sqlite3。同时,您需要创建一个名为 "house.db" 的 SQLite 数据库文件,以便在代码中进行连接和操作。
阅读全文
相关推荐
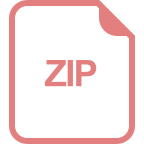


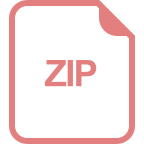
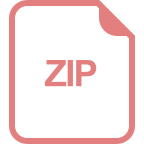
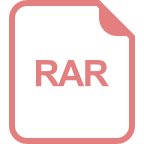
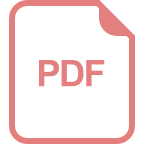

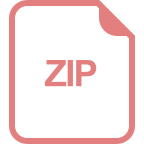
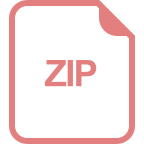
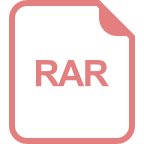
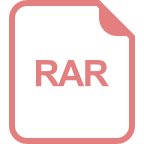
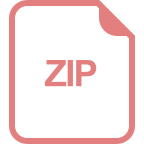
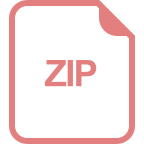
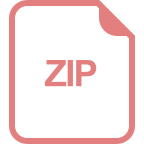