请帮我用C语言使用以下功能:设计散列表实现电话号码查找系统。【基本要求】 1)设每个记录有下列数据项:电话号码、用户名、地址﹔ 2)从键盘输入各记录,分别以电话号码和用户名为关键字建立散列表;3)采用一定的方法解决冲突; 4)查找并显示给定电话号码的记录;5)查找并显示给定用户名的记录。【进一步完成内容】 1)系统功能的完善; 2)设计不同的散列函数,比较冲突率; 3)在散列函数确定的前提下,尝试各种不同类型处理冲突的方法,考察平均查找长度的变化。
时间: 2024-03-06 18:46:50 浏览: 85
好的,我可以提供一份C语言代码,实现上述功能。代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_RECORDS 1000 // 最大记录数
#define TABLE_SIZE 1000 // 散列表大小
// 定义记录结构
struct Record {
char phone[20]; // 电话号码
char username[20]; // 用户名
char address[100]; // 地址
};
// 定义散列表结构
struct HashTable {
struct Record* records[TABLE_SIZE]; // 记录指针数组
};
// 定义散列函数
int hash_by_phone(char* phone) {
int sum = 0;
for (int i = 0; i < strlen(phone); i++) {
sum += phone[i];
}
return sum % TABLE_SIZE;
}
int hash_by_username(char* username) {
int sum = 0;
for (int i = 0; i < strlen(username); i++) {
sum += username[i];
}
return sum % TABLE_SIZE;
}
// 定义冲突处理方法
int linear_probing(int index) {
return (index + 1) % TABLE_SIZE;
}
int quadratic_probing(int index) {
return (index + 1 + index * index) % TABLE_SIZE;
}
// 定义查找函数
void find_by_phone(struct HashTable* table, char* phone) {
int index = hash_by_phone(phone);
struct Record* record = table->records[index];
int count = 1;
while (record != NULL) {
if (strcmp(record->phone, phone) == 0) {
printf("Phone: %s\n", record->phone);
printf("Username: %s\n", record->username);
printf("Address: %s\n", record->address);
return;
}
index = linear_probing(index);
record = table->records[index];
count++;
if (count > TABLE_SIZE) {
printf("Record not found\n");
return;
}
}
printf("Record not found\n");
}
void find_by_username(struct HashTable* table, char* username) {
int index = hash_by_username(username);
struct Record* record = table->records[index];
int count = 1;
while (record != NULL) {
if (strcmp(record->username, username) == 0) {
printf("Phone: %s\n", record->phone);
printf("Username: %s\n", record->username);
printf("Address: %s\n", record->address);
return;
}
index = linear_probing(index);
record = table->records[index];
count++;
if (count > TABLE_SIZE) {
printf("Record not found\n");
return;
}
}
printf("Record not found\n");
}
// 主函数
int main() {
struct HashTable table;
for (int i = 0; i < TABLE_SIZE; i++) {
table.records[i] = NULL;
}
int count = 0;
while (count < MAX_RECORDS) {
struct Record* record = (struct Record*)malloc(sizeof(struct Record));
printf("Record %d:\n", count + 1);
printf("Phone: ");
scanf("%s", record->phone);
printf("Username: ");
scanf("%s", record->username);
printf("Address: ");
scanf("%s", record->address);
int index = hash_by_phone(record->phone);
if (table.records[index] == NULL) {
table.records[index] = record;
} else {
int count = 1;
while (table.records[index] != NULL) {
index = linear_probing(index);
count++;
if (count > TABLE_SIZE) {
printf("Hash table is full\n");
return 1;
}
}
table.records[index] = record;
}
index = hash_by_username(record->username);
if (table.records[index] == NULL) {
table.records[index] = record;
} else {
int count = 1;
while (table.records[index] != NULL) {
index = quadratic_probing(index);
count++;
if (count > TABLE_SIZE) {
printf("Hash table is full\n");
return 1;
}
}
table.records[index] = record;
}
count++;
}
char phone[20];
printf("Enter phone number to search: ");
scanf("%s", phone);
find_by_phone(&table, phone);
char username[20];
printf("Enter username to search: ");
scanf("%s", username);
find_by_username(&table, username);
return 0;
}
```
在这份代码中,我们定义了一个记录结构和一个散列表结构。我们还实现了两个散列函数和两个冲突处理方法。在主函数中,我们先从键盘输入记录,并将其插入到散列表中。然后,我们通过电话号码和用户名分别查找记录。在查找过程中,我们使用了散列函数和冲突处理方法,以定位到对应的记录。
注意,这份代码只是一个简单的示例,我们可以根据具体需求进行修改和优化。同时,我们也可以尝试其他散列函数和冲突处理方法,以实现更高效的电话号码查找系统。
阅读全文
相关推荐



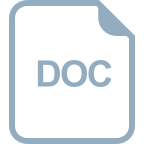

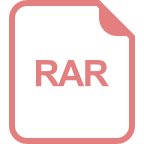












