C# httpclinet 切片上传大文件
时间: 2023-08-21 10:03:01 浏览: 108
在 C# 中使用 HttpClient 切片上传大文件,可以通过以下步骤实现:
1. 将文件切分为多个小块(切片)。你可以使用 FileStream 类来读取文件,并根据需要将文件切分为固定大小的块。
```csharp
string filePath = "your_file_path";
int chunkSize = 1024 * 1024; // 1MB
using (FileStream fileStream = new FileStream(filePath, FileMode.Open, FileAccess.Read))
{
byte[] buffer = new byte[chunkSize];
int bytesRead;
while ((bytesRead = fileStream.Read(buffer, 0, buffer.Length)) > 0)
{
// 在这里上传切片
// 可以将 buffer 作为参数传递给上传方法
}
}
```
2. 使用 HttpClient 发送每个切片到服务器。你可以使用 PostAsync 方法发送切片数据,并在请求头中指定当前切片的索引和总切片数。
```csharp
string uploadUrl = "your_upload_url";
int totalChunks = 10; // 假设文件切分为 10 个块
using (HttpClient client = new HttpClient())
{
for (int chunkIndex = 0; chunkIndex < totalChunks; chunkIndex++)
{
byte[] chunkData = GetChunkData(chunkIndex);
using (ByteArrayContent content = new ByteArrayContent(chunkData))
{
content.Headers.Add("chunk-index", chunkIndex.ToString());
content.Headers.Add("total-chunks", totalChunks.ToString());
HttpResponseMessage response = await client.PostAsync(uploadUrl, content);
// 处理响应
}
}
}
```
3. 在服务器端接收切片并进行处理。你需要在服务器端实现一个接口来处理切片上传请求,并将每个切片保存到文件中。
具体的服务器端实现取决于你使用的服务器技术和框架。你可以根据自己的需求选择适合的方式来处理切片上传请求。
这只是一个基本的框架,你可以根据自己的需求进行修改和扩展。记得在实际应用中处理错误、上传进度等方面要进行适当的处理。
阅读全文
相关推荐
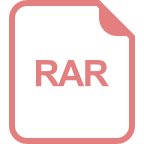
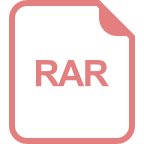
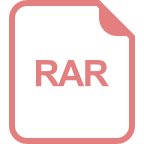
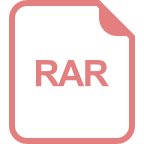
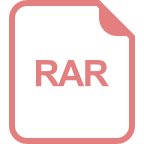




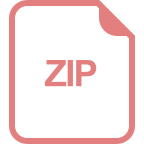
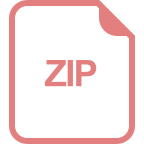
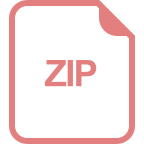
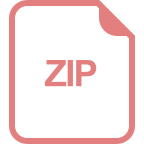