FFmpeg解码 内存泄漏
时间: 2024-06-03 09:03:24 浏览: 226
FFmpeg是一个开源的跨平台音视频处理库,它提供了丰富的功能和接口,包括解码、编码、转码、过滤等。在使用FFmpeg进行解码时,内存泄漏是一个常见的问题。
内存泄漏指的是程序在运行过程中分配的内存没有被正确释放,导致内存占用不断增加,最终可能导致程序崩溃或者系统资源耗尽。在mpeg解码过程中,内存泄漏可能出现在以下几个方面:
1. 未释放AVPacket和AVFrame:在解码过程中,AVPacket用于存储解码前的数据,AVFrame用于存储解码后的数据。如果在使用完AVPacket和AVFrame后没有正确释放它们,就会导致内存泄漏。
2. 未释放解码器上下文:解码器上下文(AVCodecContext)是用于存储解码器相关的信息和状态的结构体。如果在解码完成后没有正确释放解码器上下文,就会导致内存泄漏。
3. 未释放解码器:解码器(AVCodec)是用于实际进行解码操作的结构体。如果在使用完解码器后没有正确释放它,就会导致内存泄漏。
为了避免内存泄漏,可以采取以下几个措施:
1. 在使用完AVPacket和AVFrame后,调用相应的释放函数进行内存释放,如av_packet_unref()和av_frame_free()。
2. 在解码完成后,调用avcodec_free_context()函数释放解码器上下文。
3. 在不再需要解码器时,调用avcodec_free_context()函数释放解码器。
4. 在程序退出前,确保所有的内存都被正确释放。
相关问题
ffmpeg内存泄漏问题
FFmpeg是一个开源的跨平台音视频处理框架,它提供了丰富的API,可以用来进行音视频的编解码、转码、过滤等操作。在使用FFmpeg的过程中,由于不当的使用API或者其他原因,可能会导致内存泄漏的问题。内存泄漏是指程序在运行过程中,申请的内存没有被释放,导致内存占用不断增加,最终导致程序崩溃或者系统变慢等问题。针对FFmpeg内存泄漏问题,可以使用一些工具来帮助检查,比如vld、WinDbg、vs诊断工具等。同时,在使用FFmpeg的过程中,也需要注意API的使用方式,及时释放申请的内存,避免内存泄漏的问题的发生。
ffmpeg 解码h264视频流代码
以下是使用FFmpeg解码H.264视频流的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/time.h>
#include <sys/types.h>
#include <sys/stat.h>
extern "C"
{
#include <libavcodec/avcodec.h>
}
int main(int argc, char **argv)
{
AVCodec *codec = NULL;
AVCodecContext *codec_ctx = NULL;
AVFrame *frame = NULL;
AVPacket packet;
int ret, i, video_stream_index;
int got_frame = 0;
int frame_count = 0;
int video_width, video_height;
struct timeval start_time, end_time;
if (argc < 2)
{
printf("Usage: %s <input_file>\n", argv[0]);
return -1;
}
avcodec_register_all();
// 打开文件并读取视频流信息
AVFormatContext *format_ctx = NULL;
if (avformat_open_input(&format_ctx, argv[1], NULL, NULL) != 0)
{
printf("Couldn't open input file\n");
return -1;
}
if (avformat_find_stream_info(format_ctx, NULL) < 0)
{
printf("Couldn't find stream information\n");
return -1;
}
// 查找视频流,并初始化解码器
for (i = 0; i < format_ctx->nb_streams; i++)
{
if (format_ctx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO)
{
video_stream_index = i;
codec_ctx = avcodec_alloc_context3(codec);
avcodec_parameters_to_context(codec_ctx, format_ctx->streams[i]->codecpar);
codec = avcodec_find_decoder(codec_ctx->codec_id);
if (!codec)
{
printf("Unsupported codec\n");
return -1;
}
if (avcodec_open2(codec_ctx, codec, NULL) < 0)
{
printf("Could not open codec\n");
return -1;
}
video_width = codec_ctx->width;
video_height = codec_ctx->height;
break;
}
}
// 初始化AVFrame并分配内存
frame = av_frame_alloc();
if (!frame)
{
printf("Could not allocate frame\n");
return -1;
}
gettimeofday(&start_time, NULL);
// 读取视频流并解码
while (av_read_frame(format_ctx, &packet) >= 0)
{
if (packet.stream_index == video_stream_index)
{
ret = avcodec_decode_video2(codec_ctx, frame, &got_frame, &packet);
if (ret < 0)
{
printf("Error decoding video frame\n");
return -1;
}
if (got_frame)
{
printf("Decoded frame %d\n", frame_count++);
// 在这里可以处理解码后的帧,例如渲染到屏幕上
}
}
av_packet_unref(&packet);
}
gettimeofday(&end_time, NULL);
double elapsed_time = (end_time.tv_sec - start_time.tv_sec) + (end_time.tv_usec - start_time.tv_usec) / 1000000.0;
printf("Decoded %d frames in %f seconds (average fps: %f)\n", frame_count, elapsed_time, frame_count / elapsed_time);
avcodec_free_context(&codec_ctx);
avformat_close_input(&format_ctx);
av_frame_free(&frame);
return 0;
}
```
这段代码打开指定文件并读取视频流信息,查找视频流并初始化解码器,然后循环读取视频流并解码每个视频帧。在解码每个帧后,您可以将其渲染到屏幕上或进行其他处理。最后,它将打印解码帧的数量和解码时间,然后释放所有资源。
请注意,为了简化代码,这个示例忽略了错误处理和内存释放。在实际应用中,您需要确保正确地处理和释放所有资源,以避免内存泄漏和其他问题。
阅读全文
相关推荐
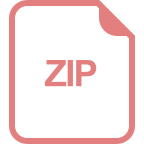
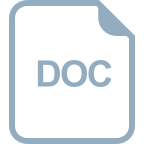
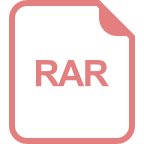
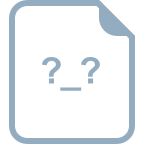
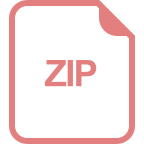
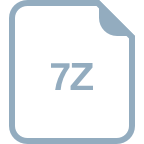
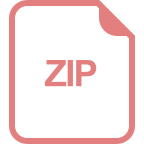
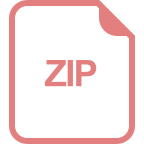
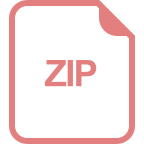

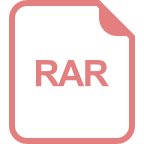
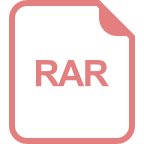
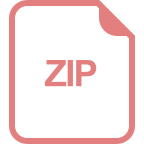
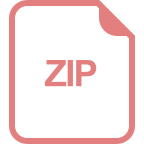
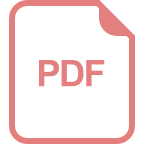