获取顺序表指定元素的位置,用c语言写代码
时间: 2024-10-07 14:04:39 浏览: 30
在C语言中,如果你有一个动态数组(如`struct Node`定义的单链表)作为顺序表,获取特定元素的位置(即索引)可以遍历整个列表来进行查找。这里是一个简单的示例,假设我们有一个结构体`Node`和一个指向头节点的指针`head`:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data; // 存储数据
struct Node* next; // 指向下一个节点
} Node;
// 获取元素位置的函数
int find_element_index(Node* head, int target) {
int index = 0;
Node* current = head;
while (current != NULL) {
if (current->data == target) {
return index; // 找到目标值,返回其索引
}
current = current->next;
index++;
}
return -1; // 如果未找到目标值,返回-1表示不在列表中
}
int main() {
// 创建并初始化链表...
int target_to_find = 5; // 需要查找的值
int element_index = find_element_index(head, target_to_find);
if (element_index != -1) {
printf("元素 %d 的位置是:%d\n", target_to_find, element_index);
} else {
printf("元素 %d 未在链表中找到\n", target_to_find);
}
return 0;
}
```
这个代码会在`main()`函数中寻找目标值,并打印出它的索引。如果没有找到,它将返回-1。
阅读全文
相关推荐
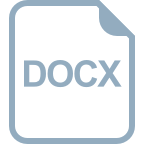
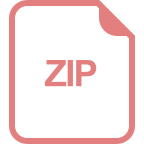
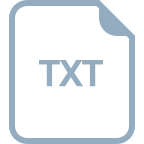
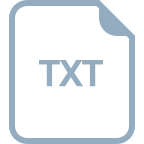
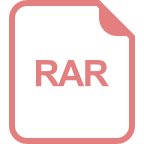
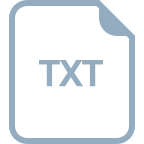
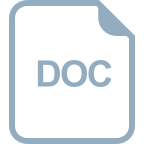
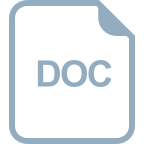
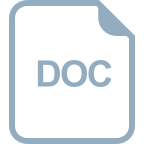
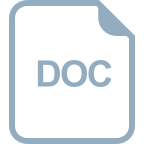
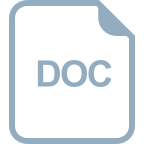
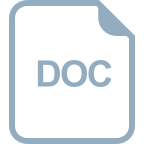
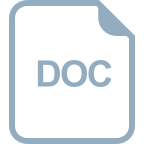





