c语言判断ip是否属于网断
时间: 2024-08-15 22:04:01 浏览: 42
在 C 语言中,判断一个 IP 地址是否属于某个网络段(子网),通常需要对 IP 地址和子网掩码进行按位与操作(`&` 操作符)。以下是一个简单的步骤:
1. **获取 IP 和子网掩码二进制表示**:
- 将 IPv4 地址转换成二进制字符串。
- 子网掩码也是一个二进制数,通常以点分十进制形式给出。例如,`255.255.255.0` 对应的二进制是 `25525525525500000000000000000000`。
2. **按位与运算**:
- 对 IP 和子网掩码做逐字节的按位与操作。比如,`IP & subnet_mask`。
3. **比较结果**:
- 如果所有字节的结果都等于 255(全 1 的状态),那么该 IP 属于该子网;如果某字节的结果为 0,则不属于。
下面是一个简化的示例代码片段:
```c
#include <stdio.h>
#include <arpa/inet.h>
int is_in_subnet(char* ip, char* subnet) {
struct in_addr ip_addr, netmask;
inet_aton(ip, &ip_addr);
inet_aton(subnet, &netmask);
uint32_t ip_int = ntohl(ip_addr.s_addr);
uint32_t netmask_int = ntohl(netmask.s_addr);
return (ip_int & netmask_int) == netmask_int;
}
int main() {
char ip[] = "192.168.1.1";
char subnet[] = "255.255.255.0";
if (is_in_subnet(ip, subnet)) {
printf("%s belongs to the subnet %s.\n", ip, subnet);
} else {
printf("%s does not belong to the subnet %s.\n", ip, subnet);
}
return 0;
}
```
阅读全文
相关推荐
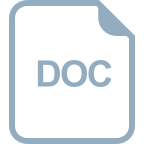
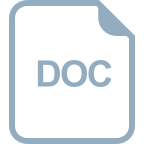
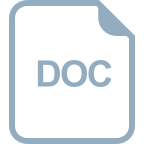
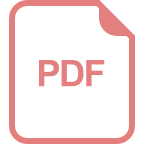
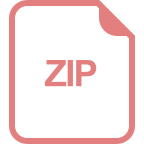
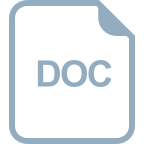
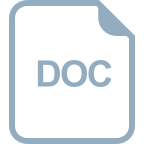
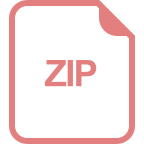
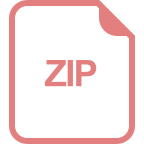
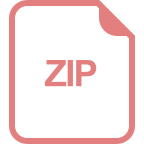
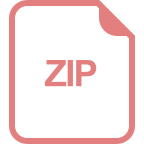
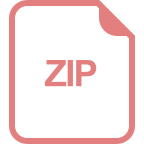
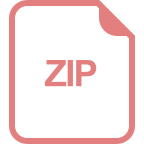