c1 = f_best_shade - f_best_traditional计算这个数列中大于0的个数
时间: 2024-12-13 20:15:46 浏览: 2
在这个表达式 `c1 = f_best_shade - f_best_traditional` 中,`f_best_shade` 和 `f_best_traditional` 可能是两个变量,其中 `f_best_shade` 表示某种类型的最好的色调(可能是某种评分或偏好),而 `f_best_traditional` 则表示传统的最佳值。如果它们都是数值类型并且 `f_best_shade` 大于 `f_best_traditional`,那么 `c1` 将是一个正数。
如果你想要计算这两个值之差为正的元素在某个列表、数组或者其他可迭代对象中的个数,你可以遍历这些元素并进行比较。以下是一个简单的Python代码片段,用于计算:
```python
# 假设 you Have 这样一个列表 values
values = [f_best_shade - f_best_traditional for i in range(len(your_list))] # 替换 your_list 为实际包含数据的列表
count_positive_diffs = sum(1 for value in values if value > 0)
```
这里,`sum(1 for value in values if value > 0)` 是一个生成器表达式,它遍历 `values` 列表,当 `value` 大于0时,生成1。`sum()` 函数则对这些1求和,得到大于0的元素个数。
相关问题
f_best_shade, f_best_traditional这两个都是一维数组,里面都有25个元素,如何计算二十五个元素对应更大的个数
要计算 `f_best_shade` 和 `f_best_traditional` 这两个一维数组中较大数值出现的次数(即哪个元素更大),你可以使用 Python 的内置函数 `enumerate()` 结合列表推导式和字典来统计每个数组中每个元素出现的次数。下面是具体的步骤:
```python
from collections import defaultdict
# 假设 f_best_shade 和 f_best_traditional 是这样的列表
f_best_shade = [value1, value2, ..., value25]
f_best_traditional = [value26, value27, ..., value50]
# 使用 defaultdict 来存储计数结果,键是元素值,值是对应的计数
count_dict_shade = defaultdict(int)
count_dict_traditional = defaultdict(int)
# 遍历两个数组并更新计数
for index, shade_value in enumerate(f_best_shade):
count_dict_shade[shade_value] += 1
for index, trad_value in enumerate(f_best_traditional):
count_dict_traditional[trad_value] += 1
# 检查哪个数组的元素出现次数更多
max_count = max(count_dict_shade.values(), count_dict_traditional.values())
best_array = 'shade' if max(count_dict_shade.values()) == max_count else 'traditional'
most_common_elements = [(key, count) for key, count in count_dict_shade.items() if count == max_count] \
+ [(key, count) for key, count in count_dict_traditional.items() if count == max_count]
print(f"The array with more occurrences: {best_array}")
print("Most common elements are:")
for element, count in most_common_elements:
print(f"{element}: {count} times")
```
这段代码首先创建了两个 defaultdict 对象,然后遍历数组并将每个元素的出现次数累加到对应的字典项中。之后找出最大值,确定哪个数组的元素更常见,并打印出最常见的元素及其出现次数。
如果你的数组不是列表形式而是 numpy 数组或其他数据结构,请告诉我,以便提供相应的处理方式。
import warnings warnings.filterwarnings("ignore") %matplotlib inline sns.distplot(train_data['V0'],fit=stats.norm) ax=plt.subplot(1,2,2) res = stats.probplot(train_data['V0'], plot=plt) ax=plt.subplot(train_rows,train_cols,i) sns.distplot(train_data[col],fit=stats.norm) res = stats.probplot(train_data[col], plot=plt) ax = sns.kdeplot(train_data['V0'], color="Red", shade=True) ax = sns.kdeplot(test_data['V0'], color="Blue", shade=True) ax = ax.legend(["train","test"]) sns.regplot(x='V0', y='target', data=train_data, ax=ax, scatter_kws={'marker': '.', 's':3, 'alpha':0.3}, line_kws={'color': 'k'} ); sns.distplot(train_data['V0'].dropna()) ax = sns.kdeplot(train_data[col], color="Red", shade=True) sns.regplot(x=col, y='target', data=train_data, ax=ax, scatter_kws={'marker':'.','s':3,'alpha':0.3}, line_kws={'color':'k'}); data_train1 = train_data.drop(['V5','V9','V11','V17','V22','V28'],axis=1) train_corr = data_train1.corr() train_corr 解释每一行代码的意思
这段代码涉及到了Python数据分析和可视化的相关库,主要作用是对训练数据进行分析和可视化。
1. `import warnings`:导入Python的警告模块。
2. `warnings.filterwarnings("ignore")`:忽略所有的警告信息。
3. `%matplotlib inline`:使用Jupyter Notebook的魔法命令,将matplotlib绘制的图形显示在notebook页面中。
4. `sns.distplot(train_data['V0'],fit=stats.norm)`:使用seaborn库绘制训练数据集中'V0'这一列的直方图,并拟合正态分布曲线。
5. `ax=plt.subplot(1,2,2)`:在当前图形中添加一个子图,子图为1行2列,当前子图为第2列。
6. `res = stats.probplot(train_data['V0'], plot=plt)`:使用scipy库绘制训练数据集中'V0'这一列的概率图。
7. `sns.distplot(train_data[col],fit=stats.norm)`:使用seaborn库绘制训练数据集中某一列的直方图,并拟合正态分布曲线。
8. `res = stats.probplot(train_data[col], plot=plt)`:使用scipy库绘制训练数据集中某一列的概率图。
9. `ax = sns.kdeplot(train_data['V0'], color="Red", shade=True)`:使用seaborn库绘制训练数据集中'V0'这一列的密度图。
10. `ax = sns.kdeplot(test_data['V0'], color="Blue", shade=True)`:使用seaborn库绘制测试数据集中'V0'这一列的密度图。
11. `ax = ax.legend(["train","test"])`:给密度图添加图例。
12. `sns.regplot(x='V0', y='target', data=train_data, ax=ax, scatter_kws={'marker': '.', 's':3, 'alpha':0.3}, line_kws={'color': 'k'})`:使用seaborn库绘制训练数据集中'V0'这一列与'target'列之间的回归关系图。
13. `sns.distplot(train_data['V0'].dropna())`:使用seaborn库绘制训练数据集中'V0'这一列的直方图,并删除缺失值。
14. `ax = sns.kdeplot(train_data[col], color="Red", shade=True)`:使用seaborn库绘制训练数据集中某一列的密度图。
15. `sns.regplot(x=col, y='target', data=train_data, ax=ax, scatter_kws={'marker':'.','s':3,'alpha':0.3}, line_kws={'color':'k'})`:使用seaborn库绘制训练数据集中某一列与'target'列之间的回归关系图。
16. `data_train1 = train_data.drop(['V5','V9','V11','V17','V22','V28'],axis=1)`:删除训练数据集中'V5'、'V9'、'V11'、'V17'、'V22'、'V28'这6列,并将处理后的数据集赋值给data_train1变量。
17. `train_corr = data_train1.corr()`:计算data_train1中各列之间的相关系数,并将结果保存到train_corr变量中。
阅读全文
相关推荐
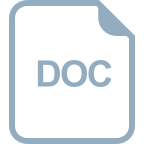
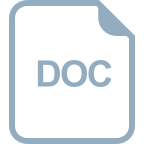
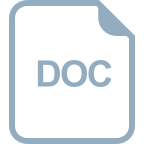
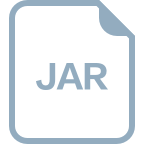
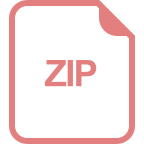
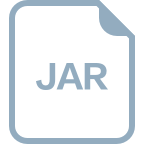
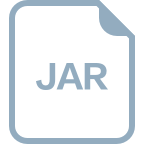
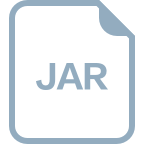
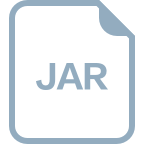
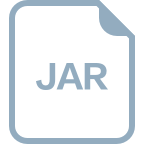
