帮我把代码注释每一步写完整
时间: 2024-12-20 20:31:45 浏览: 6
好的,我会在每个主要步骤中添加详细的注释,以便更好地理解代码的每一个部分。
```python
# 导入所需库
import numpy as np
from scipy.spatial.distance import pdist, squareform
import matplotlib.pyplot as plt
from tqdm import tqdm
# 数据提取
# 废物源数据:镇名, 经度, 纬度, 日产废物量
waste_sources = [
('石桥镇', 107.118101, 31.298319, 2.86),
('百节镇', 107.454102, 31.023069, 0.87),
# ... 其他镇 ...
('白沙镇', 108.167091, 31.967505, 0.69)
]
# 分类回收节点数据:节点名, 经度, 纬度, 固定成本, 变动成本, 排放系数, 效率
recycling_centers = [
('分类回收节点 1', 107.381715, 31.469126, 150000, 65, 7.4, 0.87),
('分类回收节点 2', 107.520675, 31.374130, 160000, 60, 6.8, 0.88),
# ... 其他节点 ...
('分类回收节点 10', 107.821522, 31.385848, 160000, 72, 7.2, 0.88)
]
# 再制造中心数据:中心名, 经度, 纬度, 固定成本, 变动成本, 排放系数, 效率
remanufacturing_centers = [
('再制造中心 1', 107.095849, 30.759173, 300000, 200, 102, 0.87),
('再制造中心 2', 107.393755, 30.881567, 305000, 210, 108, 0.86),
# ... 其他中心 ...
('再制造中心 5', 107.954275, 31.189239, 315000, 215, 102, 0.89)
]
# 填埋场数据:场名, 经度, 纬度, 变动成本, 排放系数
landfills = [
('填埋场 1', 107.063886246, 31.3623822568, 54, 6.23),
('填埋场 4', 107.92318, 31.583337, 55, 6.21),
('填埋场 7', 107.364349, 30.741412, 58, 6.32)
]
# 计算两个地点之间的欧氏距离(公里)
def haversine(lat1, lon1, lat2, lon2):
R = 6371 # 地球半径(公里)
phi1, phi2 = np.radians(lat1), np.radians(lat2) # 转换为弧度
delta_phi = np.radians(lat2 - lat1)
delta_lambda = np.radians(lon2 - lon1)
a = np.sin(delta_phi / 2) ** 2 + np.cos(phi1) * np.cos(phi2) * np.sin(delta_lambda / 2) ** 2
c = 2 * np.arctan2(np.sqrt(a), np.sqrt(1 - a))
return R * c # 返回距离
# 计算所有节点间的距离矩阵
def calculate_distances(sources, centers, landfills, manufacturing_centers):
points = []
for _, lat, lon, _ in sources:
points.append((lat, lon))
for _, lat, lon, _, _, _, _ in centers:
points.append((lat, lon))
for _, lat, lon, _, _, _, _ in manufacturing_centers:
points.append((lat, lon))
for _, lat, lon, _, _ in landfills:
points.append((lat, lon))
# 计算距离矩阵
dist = pdist(points, metric=lambda u, v: haversine(u[0], u[1], v[0], v[1]))
return squareform(dist)
# 目标函数定义
def objective_function(positions, distances, waste_sources, recycling_centers, remanufacturing_centers, landfills):
n_sources = len(waste_sources)
n_centers = len(recycling_centers)
n_manu_centers = len(remanufacturing_centers)
n_landfills = len(landfills)
source_positions = positions[:n_sources].astype(int)
center_positions = positions[n_sources:n_sources + n_centers].astype(int)
manu_center_positions = positions[n_sources + n_centers:-n_landfills].astype(int)
landfill_positions = positions[-n_landfills:].astype(int)
total_cost = 0
total_emission = 0
alpha = 5.5 # 单位距离运输成本(元/吨•千米)
beta = 0.35 # 单位距离运输碳排放因子(kg/t•km)
for i in range(n_sources):
source_id = source_positions[i]
source_waste = waste_sources[i][3]
for j in range(n_centers):
if center_positions[j] == source_id:
rec_id = j
rec_fixed_cost = recycling_centers[j][3]
rec_variable_cost = recycling_centers[j][4]
rec_emission = recycling_centers[j][5]
transport_distance = distances[i][j + n_sources]
transport_cost = alpha * source_waste * transport_distance
transport_emission = beta * source_waste * transport_distance
total_cost += rec_fixed_cost + rec_variable_cost * source_waste + transport_cost
total_emission += rec_emission * source_waste + transport_emission
rec_waste_to_manu = source_waste * 0.5
rec_waste_to_landfill = source_waste * 0.5
for k in range(n_manu_centers):
if manu_center_positions[k] == rec_id:
manu_fixed_cost = remanufacturing_centers[k][3]
manu_variable_cost = remanufacturing_centers[k][4]
manu_emission = remanufacturing_centers[k][5]
transport_distance_to_manu = distances[j + n_sources][k + n_sources + n_centers]
transport_cost_to_manu = alpha * rec_waste_to_manu * transport_distance_to_manu
transport_emission_to_manu = beta * rec_waste_to_manu * transport_distance_to_manu
total_cost += manu_fixed_cost + manu_variable_cost * rec_waste_to_manu + transport_cost_to_manu
total_emission += manu_emission * rec_waste_to_manu + transport_emission_to_manu
break
for l in range(n_landfills):
if landfill_positions[l] == rec_id:
landfill_variable_cost = landfills[l][3]
landfill_emission = landfills[l][4]
transport_distance_to_landfill = distances[j + n_sources][l + n_sources + n_centers + n_manu_centers]
transport_cost_to_landfill = alpha * rec_waste_to_landfill * transport_distance_to_landfill
transport_emission_to_landfill = beta * rec_waste_to_landfill * transport_distance_to_landfill
total_cost += landfill_variable_cost * rec_waste_to_landfill + transport_cost_to_landfill
total_emission += landfill_emission * rec_waste_to_landfill + transport_emission_to_landfill
break
return total_cost, total_emission
# PSO 初始化
def initialize_population(num_particles, num_dimensions):
particles = np.random.rand(num_particles, num_dimensions) # 初始粒子位置
velocities = np.zeros_like(particles) # 初始粒子速度
personal_best_positions = particles.copy() # 初始个人最优位置
personal_best_values = np.full(num_particles, float('inf')) # 初始个人最优值
global_best_position = None # 初始全局最优位置
global_best_value = float('inf') # 初始全局最优值
return particles, velocities, personal_best_positions, personal_best_values, global_best_position, global_best_value
# 更新个人最佳和全局最佳
def update_best_positions(values, particles, personal_best_values, personal_best_positions, global_best_value, global_best_position):
for i, value in enumerate(values):
if value < personal_best_values[i]:
personal_best_positions[i] = particles[i]
personal_best_values[i] = value
global_best_value_new = np.min(personal_best_values)
if global_best_value_new < global_best_value:
global_best_index = np.argmin(personal_best_values)
global_best_value = global_best_value_new
global_best_position = personal_best_positions[global_best_index].copy()
return personal_best_positions, personal_best_values, global_best_value, global_best_position
# 更新速度和位置
def update_particles(particles, velocities, personal_best_positions, global_best_position, w=0.5, c1=2, c2=2):
r1 = np.random.rand(*particles.shape)
r2 = np.random.rand(*particles.shape)
velocities = w * velocities + c1 * r1 * (personal_best_positions - particles) + c2 * r2 * (global_best_position - particles)
particles += velocities
return particles, velocities
# 主程序
def pso_main(distances, waste_sources, recycling_centers, remanufacturing_centers, landfills, max_iterations=100, num_particles=30, verbose=True):
num_dimensions = len(waste_sources) + len(recycling_centers) + len(remanufacturing_centers) + len(landfills)
particles, velocities, personal_best_positions, personal_best_values, global_best_position, global_best_value = initialize_population(num_particles, num_dimensions)
best_costs = []
best_emissions = []
for iteration in tqdm(range(max_iterations)):
costs, emissions = zip(*[objective_function(p, distances, waste_sources, recycling_centers, remanufacturing_centers, landfills) for p in particles])
personal_best_positions, personal_best_values, global_best_value, global_best_position = update_best_positions(costs, particles, personal_best_values, personal_best_positions, global_best_value, global_best_position)
best_costs.append(min(costs))
best_emissions.append(min(emissions))
particles, velocities = update_particles(particles, velocities, personal_best_positions, global_best_position)
if verbose:
print(f'Iteration {iteration}: Best Cost: {best_costs[-1]}, Best Emission: {best_emissions[-1]}')
return best_costs, best_emissions, global_best_position
if __name__ == "__main__":
distances = calculate_distances(waste_sources, recycling_centers, landfills, remanufacturing_centers)
best_costs, best_emissions, global_best_position = pso_main(distances, waste_sources, recycling_centers, remanufacturing_centers, landfills)
# 绘制收敛曲线
fig, ax1 = plt.subplots()
ax1.plot(best_costs, 'r-', label='Cost')
ax1.set_xlabel('Iterations')
ax1.set_ylabel('Total Cost')
ax2 = ax1.twinx()
ax2.plot(best_emissions, 'b-', label='Emission')
ax2.set_ylabel('Total Emission')
plt.title('Convergence of PSO Algorithm')
fig.tight_layout()
plt.show()
```
### 注释解释
1. **导入库**:导入所需的数学计算库 `numpy`,距离计算库 `scipy.spatial.distance` 和绘图库 `matplotlib.pyplot`,以及进度条库 `tqdm`。
2. **数据提取**:定义废物源、分类回收节点、再制造中心和填埋场的数据。
3. **距离计算**:定义 `haversine` 函数来计算两点间的欧氏距离,并使用 `pdist` 和 `squareform` 生成距离矩阵。
4. **目标函数**:定义 `objective_function` 函数来计算总成本和总排放量。
5. **PSO 初始化**:定义 `initialize_population` 函数来初始化粒子群优化算法的参数。
6. **更新个人最佳和全局最佳**:定义 `update_best_positions` 函数来更新每个粒子的个人最佳位置和全局最佳位置。
7. **更新速度和位置**:定义 `update_particles` 函数来更新粒子的速度和位置。
8. **主程序**:定义 `pso_main` 函数来执行粒子群优化算法的核心逻辑,并记录每次迭代的最佳结果。
9. **绘制收敛曲线**:在主程序执行完成后,绘制成本和排放量的收敛曲线图。
希望这些注释能帮助您更好地理解代码的每一部分!
阅读全文
相关推荐
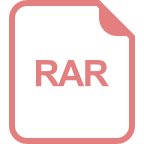
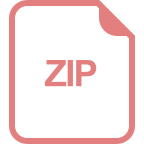
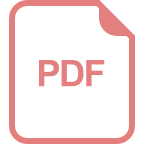
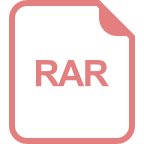
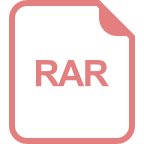
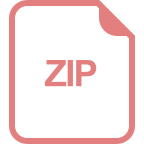
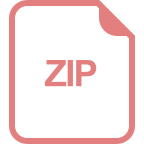
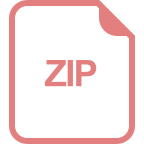
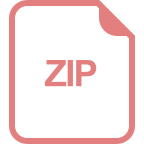
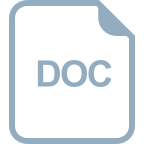
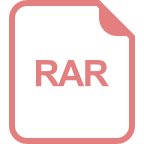
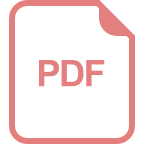
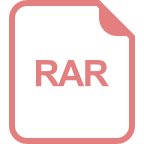
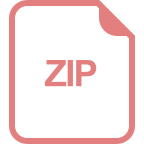
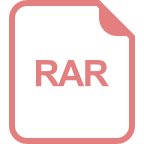
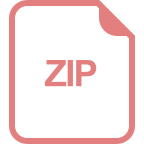
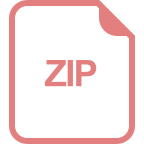
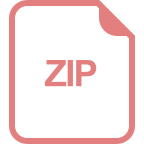
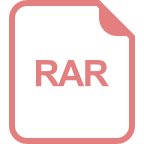