新建springboot整合redis项目
时间: 2024-04-20 16:20:13 浏览: 90
当你新建一个Spring Boot项目并整合Redis时,你需要按照以下步骤进行操作:
1. 首先,你需要创建一个新的Spring Boot项目。你可以使用Spring Initializr(https://start.spring.io/)来生成一个基本的Spring Boot项目结构。
2. 在生成的项目中,你需要添加Redis的依赖。在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
3. 接下来,你需要配置Redis连接信息。在application.properties(或application.yml)文件中添加以下配置:
```properties
spring.redis.host=your_redis_host
spring.redis.port=your_redis_port
spring.redis.password=your_redis_password (如果有密码的话)
```
4. 现在,你可以在你的代码中使用Redis了。你可以通过注入`RedisTemplate`或者`StringRedisTemplate`来操作Redis。例如,你可以在一个Service类中注入`StringRedisTemplate`并使用它来进行操作:
```java
@Service
public class RedisService {
@Autowired
private StringRedisTemplate redisTemplate;
public void setValue(String key, String value) {
redisTemplate.opsForValue().set(key, value);
}
public String getValue(String key) {
return redisTemplate.opsForValue().get(key);
}
}
```
5. 最后,你可以在你的应用程序中使用`RedisService`来进行Redis操作。例如,在一个Controller类中使用`RedisService`:
```java
@RestController
public class RedisController {
@Autowired
private RedisService redisService;
@GetMapping("/redis/{key}")
public String getValue(@PathVariable String key) {
return redisService.getValue(key);
}
@PostMapping("/redis")
public void setValue(@RequestParam String key, @RequestParam String value) {
redisService.setValue(key, value);
}
}
```
这样,你就成功地创建了一个Spring Boot项目并整合了Redis。
阅读全文
相关推荐
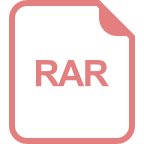
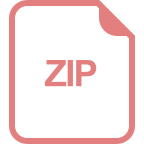
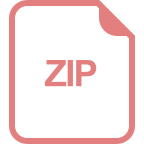
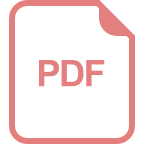

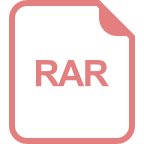
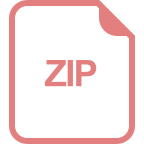
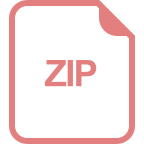
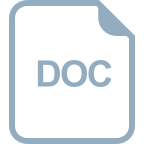
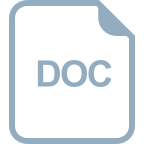
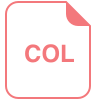

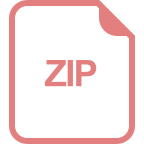
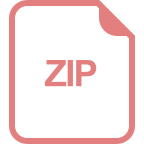
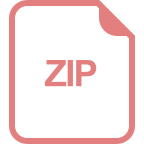
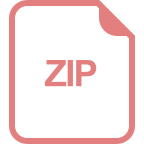