#include<iostream> #include<fstream> using namespace std; int main() { fstream in; char buffer[100]; in.open("12.cpp",ios::in); fstream out("12.txt",ios::out); int line_nume=0; while(!in.eof())//若读到文件尾部才结束 { in.getline(buffer,100); ++line_nume; out<<line_nume<<":"<<buffer<<endl; cout<<line_nume<<":"<<buffer<<endl;//输出所读的文件内容 } in.close(); out.close(); return 0; } 优化这段代码
时间: 2024-01-21 17:16:48 浏览: 26
这段代码可以进行以下优化:
1. 使用 const string 定义文件名,避免在多个地方使用字符串字面量,可读性更好。
2. 使用 std::string 类型代替字符数组 buffer,更加安全。
3. 在 while 循环中使用 getline(),避免出现读取行数不对的情况。
4. 将文件打开和关闭的操作移到 while 循环外部,避免重复操作,提高效率。
5. 使用 ++line_num 代替 line_num++,提高效率。
6. 增加错误处理,比如文件打开失败后应该进行错误提示。
改进后的代码如下:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
const string FILE_NAME = "12.cpp";
int main() {
fstream in;
string buffer;
in.open(FILE_NAME, ios::in);
if (!in.is_open()) {
cerr << "Failed to open file: " << FILE_NAME << endl;
return 1;
}
fstream out("12.txt", ios::out);
int line_num = 0;
while (getline(in, buffer)) {
++line_num;
out << line_num << ":" << buffer << endl;
cout << line_num << ":" << buffer << endl;
}
in.close();
out.close();
return 0;
}
```
相关推荐
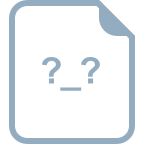














