帮我用c语言做一个银行管理系统
时间: 2023-05-11 19:03:20 浏览: 46
好的,我可以为您提供一个基本的银行管理系统的代码框架,您可以在此基础上进行修改和完善。以下是代码:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_ACCOUNTS 1000
#define MAX_NAME_LENGTH 50
#define MAX_PASSWORD_LENGTH 20
typedef struct {
int account_number;
char name[MAX_NAME_LENGTH];
char password[MAX_PASSWORD_LENGTH];
double balance;
} Account;
Account accounts[MAX_ACCOUNTS];
int num_accounts = 0;
void create_account() {
if (num_accounts >= MAX_ACCOUNTS) {
printf("Error: Maximum number of accounts reached.\n");
return;
}
Account new_account;
printf("Enter account number: ");
scanf("%d", &new_account.account_number);
for (int i = 0; i < num_accounts; i++) {
if (accounts[i].account_number == new_account.account_number) {
printf("Error: Account number already exists.\n");
return;
}
}
printf("Enter name: ");
scanf("%s", new_account.name);
printf("Enter password: ");
scanf("%s", new_account.password);
printf("Enter initial balance: ");
scanf("%lf", &new_account.balance);
accounts[num_accounts] = new_account;
num_accounts++;
printf("Account created successfully.\n");
}
void deposit() {
int account_number;
printf("Enter account number: ");
scanf("%d", &account_number);
int account_index = -1;
for (int i = 0; i < num_accounts; i++) {
if (accounts[i].account_number == account_number) {
account_index = i;
break;
}
}
if (account_index == -1) {
printf("Error: Account not found.\n");
return;
}
char password[MAX_PASSWORD_LENGTH];
printf("Enter password: ");
scanf("%s", password);
if (strcmp(password, accounts[account_index].password) != 0) {
printf("Error: Incorrect password.\n");
return;
}
double amount;
printf("Enter amount to deposit: ");
scanf("%lf", &amount);
accounts[account_index].balance += amount;
printf("Deposit successful. New balance: %.2lf\n", accounts[account_index].balance);
}
void withdraw() {
int account_number;
printf("Enter account number: ");
scanf("%d", &account_number);
int account_index = -1;
for (int i = 0; i < num_accounts; i++) {
if (accounts[i].account_number == account_number) {
account_index = i;
break;
}
}
if (account_index == -1) {
printf("Error: Account not found.\n");
return;
}
char password[MAX_PASSWORD_LENGTH];
printf("Enter password: ");
scanf("%s", password);
if (strcmp(password, accounts[account_index].password) != 0) {
printf("Error: Incorrect password.\n");
return;
}
double amount;
printf("Enter amount to withdraw: ");
scanf("%lf", &amount);
if (amount > accounts[account_index].balance) {
printf("Error: Insufficient balance.\n");
return;
}
accounts[account_index].balance -= amount;
printf("Withdrawal successful. New balance: %.2lf\n", accounts[account_index].balance);
}
void display_balance() {
int account_number;
printf("Enter account number: ");
scanf("%d", &account_number);
int account_index = -1;
for (int i = 0; i < num_accounts; i++) {
if (accounts[i].account_number == account_number) {
account_index = i;
break;
}
}
if (account_index == -1) {
printf("Error: Account not found.\n");
return;
}
char password[MAX_PASSWORD_LENGTH];
printf("Enter password: ");
scanf("%s", password);
if (strcmp(password, accounts[account_index].password) != 0) {
printf("Error: Incorrect password.\n");
return;
}
printf("Account balance: %.2lf\n", accounts[account_index].balance);
}
int main() {
int choice;
while (1) {
printf("1. Create account\n");
printf("2. Deposit\n");
printf("3. Withdraw\n");
printf("4. Display balance\n");
printf("5. Exit\n");
printf("Enter choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
create_account();
break;
case 2:
deposit();
break;
case 3:
withdraw();
break;
case 4:
display_balance();
break;
case 5:
exit(0);
default:
printf("Invalid choice.\n");
}
}
return 0;
}
相关推荐
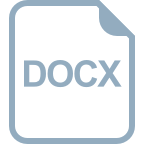
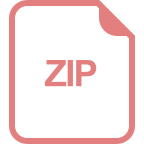














