编写一个马里奥,和一个墙体,实现马里奥能站到墙上,并且不能穿墙
时间: 2024-06-08 10:10:40 浏览: 11
这里给出一个简单的方案,使用Unity游戏引擎实现。
首先,我们创建一个马里奥模型和一个墙体模型。然后创建两个脚本:一个是控制马里奥运动的脚本,一个是控制墙体碰撞的脚本。
在控制马里奥运动的脚本中,我们需要检测马里奥是否接触到了墙体,如果接触到了,就让马里奥停止运动。具体实现如下:
```csharp
public class MarioController : MonoBehaviour
{
public float speed = 5f;
public float jumpForce = 10f;
public Transform groundCheck;
public LayerMask groundLayer;
public float groundCheckRadius = 0.1f;
private Rigidbody2D rb;
private bool isGrounded;
private bool isTouchingWall;
private bool isFacingRight = true;
void Start()
{
rb = GetComponent<Rigidbody2D>();
}
void FixedUpdate()
{
//检测是否在地面上
isGrounded = Physics2D.OverlapCircle(groundCheck.position, groundCheckRadius, groundLayer);
//获取水平输入
float moveInput = Input.GetAxis("Horizontal");
//移动马里奥
rb.velocity = new Vector2(moveInput * speed, rb.velocity.y);
//转向
if (moveInput > 0 && !isFacingRight)
{
Flip();
}
else if (moveInput < 0 && isFacingRight)
{
Flip();
}
//跳跃
if (Input.GetKeyDown(KeyCode.Space) && isGrounded)
{
rb.velocity = new Vector2(rb.velocity.x, jumpForce);
}
}
void OnCollisionEnter2D(Collision2D collision)
{
//检测是否碰到了墙体
if (collision.gameObject.CompareTag("Wall"))
{
isTouchingWall = true;
rb.velocity = Vector2.zero;
}
}
void OnCollisionExit2D(Collision2D collision)
{
//检测是否离开了墙体
if (collision.gameObject.CompareTag("Wall"))
{
isTouchingWall = false;
}
}
void Flip()
{
isFacingRight = !isFacingRight;
transform.localScale = new Vector3(-transform.localScale.x, transform.localScale.y, transform.localScale.z);
}
}
```
在控制墙体碰撞的脚本中,我们需要检测马里奥是否碰到了墙体,并且让马里奥不能穿墙。具体实现如下:
```csharp
public class WallController : MonoBehaviour
{
private void OnCollisionEnter2D(Collision2D collision)
{
//检测是否碰到了马里奥
if (collision.gameObject.CompareTag("Player"))
{
//检测马里奥是否在墙体左边或右边
float playerPosX = collision.gameObject.transform.position.x;
float wallPosX = transform.position.x;
float distance = Mathf.Abs(playerPosX - wallPosX);
float playerWidth = collision.gameObject.GetComponent<SpriteRenderer>().bounds.size.x / 2;
if (distance < playerWidth)
{
//马里奥不能穿墙
float pushForce = 10f;
if (playerPosX < wallPosX)
{
collision.gameObject.GetComponent<Rigidbody2D>().AddForce(new Vector2(-pushForce, 0f), ForceMode2D.Impulse);
}
else
{
collision.gameObject.GetComponent<Rigidbody2D>().AddForce(new Vector2(pushForce, 0f), ForceMode2D.Impulse);
}
}
}
}
}
```
将这两个脚本分别挂载到马里奥和墙体上,然后在场景中添加马里奥和墙体,就可以实现马里奥能站到墙上,并且不能穿墙的效果了。
相关推荐





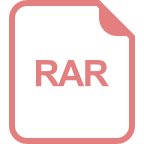
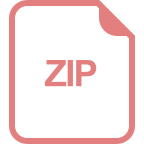
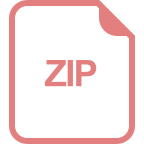
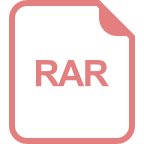
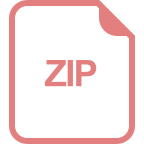