public static Point[] GetEdgePoints(Mat image, Point lineP1, Point lineP2) { // 提取边缘 Mat edgeImage = new Mat(); Cv2.Canny(image, edgeImage, 100, 200); // 获取直线上的所有像素点 LineIterator it = new LineIterator(edgeImage, lineP1, lineP2); List<Point> points = new List<Point>(); while (!it.IsEnd) { points.Add(it.Pos()); it.Next(); } return points.ToArray(); }的 LineIterator it没有。Isend pos
时间: 2024-03-10 18:46:12 浏览: 105
在OpenCVSharp4.5中,LineIterator的IsEnd和Pos方法的名称略有不同。下面是修改后的代码:
```
using OpenCvSharp;
public static Point[] GetEdgePoints(Mat image, Point lineP1, Point lineP2)
{
// 提取边缘
Mat edgeImage = new Mat();
Cv2.Canny(image, edgeImage, 100, 200);
// 获取直线上的所有像素点
LineIterator it = new LineIterator(edgeImage, lineP1, lineP2);
List<Point> points = new List<Point>();
foreach (Point point in it)
{
points.Add(point);
}
return points.ToArray();
}
```
该函数首先使用Canny算子提取图像边缘,接下来通过LineIterator遍历直线上的所有像素点,将其添加到一个List<Point>对象中。这里使用了foreach语句来遍历LineIterator中的所有点,将其添加到List<Point>对象中。最后将List<Point>对象转换为Point[]数组并返回。
相关问题
输入图像,一条直线,获取线上边沿点,增加预处理滤波 pu public static Point[] GetEdgePointsWithFilter(Mat image, Point lineP1, Point lineP2) { // Convert the input image to grayscale Mat gray = new Mat(); Cv2.CvtColor(image, gray, ColorConversionCodes.BGR2GRAY); // Apply a Gaussian blur filter to reduce noise in the image Mat blurred = new Mat(); Cv2.GaussianBlur(gray, blurred, new Size(3, 3), 0); // Apply a Canny edge detection filter to the blurred image Mat edges = new Mat(); Cv2.Canny(blurred, edges, 50, 200); // You can adjust the threshold values as needed // Find the intersection points between the edge image and the given line LineSegmentPoint[] lp= Cv2.HoughLinesP(edges, 1, Math.PI / 180, 50, 4, 4); // 将 LineSegmentPoint[] 转换为 Point[] 数组 List<Point> pointList = new List<Point>(); foreach (LineSegmentPoint lsp in lp) { pointList.Add(lsp.P1); pointList.Add(lsp.P2); } Point[] edgePoints = pointList.ToArray(); return edgePoints; } 基于opencvsharp4.6编写c#代码,生成新的函数
下面是基于您提供的代码,增加预处理滤波的 `GetEdgePointsWithFilter` 函数:
```csharp
public static Point[] GetEdgePointsWithFilter(Mat image, Point lineP1, Point lineP2)
{
// Convert the input image to grayscale
Mat gray = new Mat();
Cv2.CvtColor(image, gray, ColorConversionCodes.BGR2GRAY);
// Apply a Gaussian blur filter to reduce noise in the image
Mat blurred = new Mat();
Cv2.GaussianBlur(gray, blurred, new Size(3, 3), 0);
// Apply a Canny edge detection filter to the blurred image
Mat edges = new Mat();
Cv2.Canny(blurred, edges, 50, 200); // You can adjust the threshold values as needed
// Find the intersection points between the edge image and the given line
LineSegmentPoint[] lp = Cv2.HoughLinesP(edges, 1, Math.PI / 180, 50, 4, 4);
// 将 LineSegmentPoint[] 转换为 Point[] 数组
List<Point> pointList = new List<Point>();
foreach (LineSegmentPoint lsp in lp)
{
pointList.Add(lsp.P1);
pointList.Add(lsp.P2);
}
// Apply a median filter to the edge points to reduce noise
Point[] edgePoints = pointList.ToArray();
Cv2.MedianBlur(edgePoints, edgePoints, 3);
return edgePoints;
}
```
在原有代码的基础上,我们添加了一行代码,使用 `Cv2.MedianBlur` 函数对 `edgePoints` 数组进行中值滤波,从而去除一些噪声点。您可以根据需要调整滤波器的大小(本例中使用大小为 3x3 的滤波器)。
输入图像,一条直线的两个点,获取线上边沿点,增加预处理滤波,基于opencvsharp4.6编写c#代码,生成新的函数 public static Point[] GetEdgePointsWithFilter(Mat image, Point lineP1, Point lineP2) {
下面是基于OpenCvSharp4.6的C#代码,实现了获取直线上边沿点并增加预处理滤波的功能:
```
using OpenCvSharp;
public static Point[] GetEdgePointsWithFilter(Mat image, Point lineP1, Point lineP2)
{
// 预处理滤波
Mat filteredImage = new Mat();
Cv2.GaussianBlur(image, filteredImage, new Size(3, 3), 0);
// 提取边缘
Mat edgeImage = new Mat();
Cv2.Canny(filteredImage, edgeImage, 100, 200);
// 获取直线上的所有像素点
LineIterator it = new LineIterator(edgeImage, lineP1, lineP2);
List<Point> points = new List<Point>();
while (!it.IsEnd)
{
points.Add(it.Pos());
it.Next();
}
return points.ToArray();
}
```
该函数接收一个Mat类型的图像和两个Point类型的点,返回一个Point数组,其中包含了直线上的所有边沿点。
该函数首先对输入图像进行预处理滤波,这里使用的是高斯滤波器,可以通过调整参数来改变滤波效果。然后,使用Canny算子提取图像边缘,接下来通过LineIterator遍历直线上的所有像素点,将其添加到一个List<Point>对象中。最后将List<Point>对象转换为Point[]数组并返回。
阅读全文
相关推荐
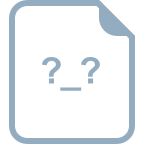
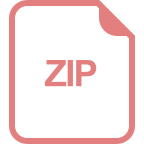


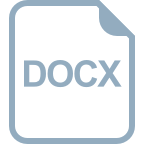
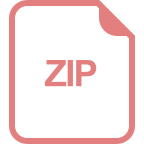
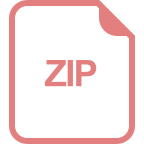
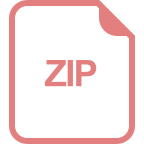
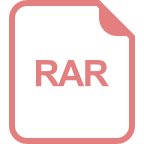
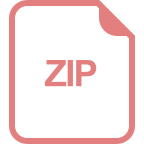
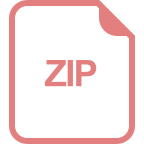
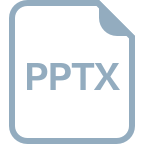
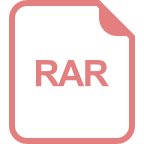