请用springboot和vue写出一个前后端分离websocekt的demo项目
时间: 2023-12-15 17:07:33 浏览: 85
好的,下面是一个简单的前后端分离websocekt的demo项目,使用SpringBoot作为后端框架,Vue作为前端框架。
1. 后端代码
在SpringBoot中,我们使用Spring WebSocket模块来实现WebSocket功能。首先,我们需要添加以下依赖到我们的pom.xml文件中:
```
<!-- Spring WebSocket -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
```
然后,在我们的SpringBoot主类中,添加@EnableWebSocket注解,启用WebSocket功能:
```
@SpringBootApplication
@EnableWebSocket
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
接下来,我们创建一个WebSocket处理器类,处理WebSocket连接的建立、关闭和消息发送:
```
@Component
public class WebSocketHandler extends TextWebSocketHandler {
private static final Logger LOGGER = LoggerFactory.getLogger(WebSocketHandler.class);
private List<WebSocketSession> sessions = new ArrayList<>();
@Override
public void afterConnectionEstablished(WebSocketSession session) throws Exception {
LOGGER.info("WebSocket session opened: " + session.getId());
sessions.add(session);
}
@Override
protected void handleTextMessage(WebSocketSession session, TextMessage message) throws Exception {
LOGGER.info("Received message: " + message.getPayload());
for (WebSocketSession s : sessions) {
s.sendMessage(message);
}
}
@Override
public void afterConnectionClosed(WebSocketSession session, CloseStatus status) throws Exception {
LOGGER.info("WebSocket session closed: " + session.getId());
sessions.remove(session);
}
}
```
这个处理器类继承自TextWebSocketHandler,重写了三个方法:
- afterConnectionEstablished:当WebSocket连接建立时调用,将当前连接添加到sessions列表中;
- handleTextMessage:当收到文本消息时调用,遍历sessions列表,向所有连接发送消息;
- afterConnectionClosed:当WebSocket连接关闭时调用,将当前连接从sessions列表中移除。
最后,我们在配置类中添加WebSocket配置:
```
@Configuration
@EnableWebSocket
public class WebSocketConfig implements WebSocketConfigurer {
@Autowired
private WebSocketHandler webSocketHandler;
@Override
public void registerWebSocketHandlers(WebSocketHandlerRegistry registry) {
registry.addHandler(webSocketHandler, "/websocket").setAllowedOrigins("*");
}
}
```
这个配置类使用@Autowired注解注入WebSocket处理器,并将处理器注册到“/websocket”路径下。
2. 前端代码
在Vue中,我们使用Vue-WebSocket插件来实现WebSocket功能。首先,我们需要使用npm安装Vue-WebSocket插件:
```
npm install --save vue-native-websocket
```
然后,在我们的Vue组件中,引入Vue-WebSocket插件,连接WebSocket服务器:
```
<template>
<div>
<input type="text" v-model="message">
<button @click="send">Send</button>
<ul>
<li v-for="msg in messages">{{ msg }}</li>
</ul>
</div>
</template>
<script>
import VueNativeSock from 'vue-native-websocket'
export default {
data() {
return {
message: '',
messages: []
}
},
mounted() {
this.$connect('ws://localhost:8080/websocket')
this.$on('message', message => {
this.messages.push(message.data)
})
},
methods: {
send() {
this.$socket.send(this.message)
this.message = ''
}
},
destroyed() {
this.$disconnect()
},
sockets: {
open() {
console.log('WebSocket connected')
},
close() {
console.log('WebSocket disconnected')
}
}
}
</script>
```
这个Vue组件使用VueNativeSock插件连接到“ws://localhost:8080/websocket”路径下的WebSocket服务器,并监听“message”事件,将收到的消息添加到messages数组中。同时,它也提供了一个输入框和一个发送按钮,点击发送按钮后,将输入框中的内容发送给服务器。
3. 整合前后端代码
我们将后端代码打包成jar包,并启动服务器。然后,在Vue项目中,使用vue-cli-service serve命令启动开发服务器,将Vue组件打包成前端页面。
在前端页面中,我们使用WebSocket连接到后端服务器,并通过Vue-WebSocket插件监听WebSocket消息。当用户输入消息并点击发送时,将消息发送到后端服务器,后端服务器将消息发送给所有连接的客户端。
这样,我们就完成了一个简单的前后端分离WebSocket的demo项目。
阅读全文
相关推荐
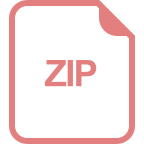
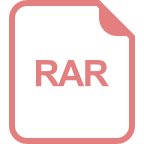
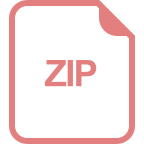
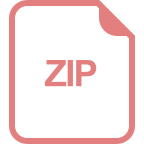
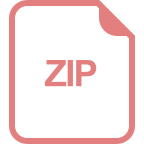
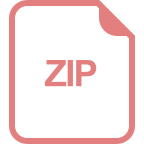
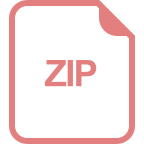
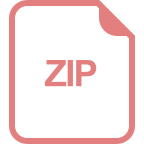
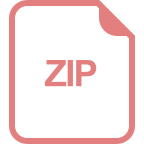
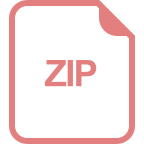
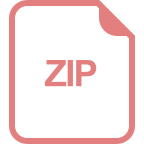
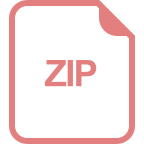
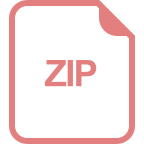
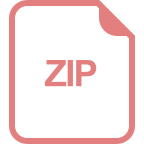
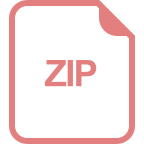
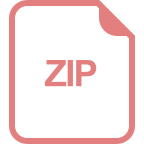
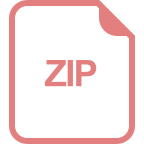
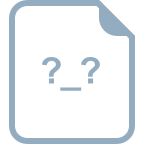
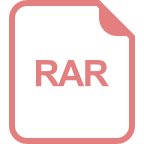