在VS2019上用C#写一个模拟时间片轮转法,输出为就绪队列正在处理某个进程,输入队列正在处理某个进程,输出队列正在处理某个进程,其他队列正在处理某个进程,以上4个队列并行处理进程
时间: 2023-12-15 15:06:20 浏览: 51
以下是一个简单的模拟时间片轮转法的 C# 代码实现:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace TimeSlice
{
class Program
{
static void Main(string[] args)
{
// 初始化就绪队列、输入队列、输出队列和其他队列
List<int> readyQueue = new List<int>();
List<int> inputQueue = new List<int>();
List<int> outputQueue = new List<int>();
List<int> otherQueue = new List<int>();
// 将进程加入就绪队列
readyQueue.Add(1);
readyQueue.Add(2);
readyQueue.Add(3);
readyQueue.Add(4);
readyQueue.Add(5);
// 模拟时间片轮转法
int timeSlice = 2;
while (readyQueue.Count > 0 || inputQueue.Count > 0 || outputQueue.Count > 0 || otherQueue.Count > 0)
{
// 就绪队列处理进程
if (readyQueue.Count > 0)
{
int processId = readyQueue[0];
Console.WriteLine("就绪队列正在处理进程 " + processId);
readyQueue.RemoveAt(0);
timeSlice--;
if (timeSlice == 0)
{
timeSlice = 2;
readyQueue.Add(processId);
}
}
// 输入队列处理进程
if (inputQueue.Count > 0)
{
int processId = inputQueue[0];
Console.WriteLine("输入队列正在处理进程 " + processId);
inputQueue.RemoveAt(0);
}
// 输出队列处理进程
if (outputQueue.Count > 0)
{
int processId = outputQueue[0];
Console.WriteLine("输出队列正在处理进程 " + processId);
outputQueue.RemoveAt(0);
}
// 其他队列处理进程
if (otherQueue.Count > 0)
{
int processId = otherQueue[0];
Console.WriteLine("其他队列正在处理进程 " + processId);
otherQueue.RemoveAt(0);
}
}
}
}
}
```
该代码中,我们初始化了就绪队列、输入队列、输出队列和其他队列,并将一些进程加入到就绪队列中。然后,我们模拟了时间片轮转法的执行过程,即不断轮流处理就绪队列、输入队列、输出队列和其他队列中的进程,直到所有队列中的进程都被处理完毕。
在处理每个队列中的进程时,我们首先判断该队列是否为空,如果不为空,则取出队首进程进行处理,并输出相应的提示信息。同时,我们使用一个计数器来模拟时间片轮转的时间片,当计数器为 0 时,将当前处理的进程重新加入到就绪队列中。
需要注意的是,该代码只是一个简单的模拟实现,实际应用中可能需要更加复杂的算法来处理不同优先级的进程、防止死锁等问题。
阅读全文
相关推荐
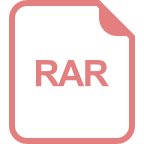


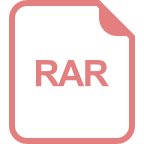
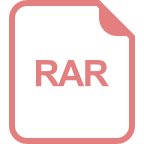
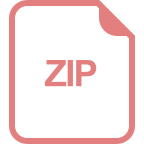
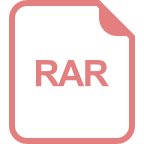
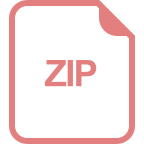
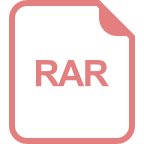
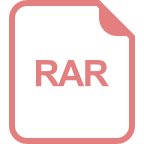
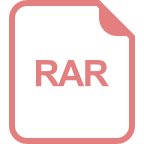
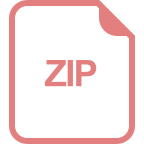
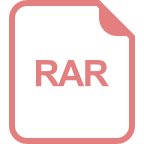
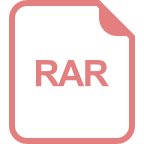
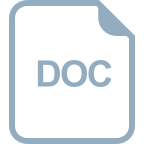
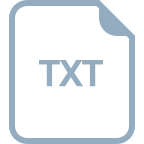
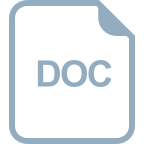