蚁群算法路线规划代码
时间: 2024-06-23 12:01:07 浏览: 218
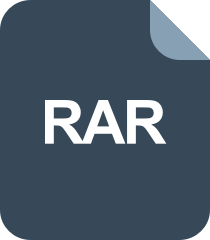
蚁群算法路径规划
蚁群算法(Ant Colony Optimization, ACO)是一种模拟蚂蚁寻找食物路径的优化搜索方法,常用于解决复杂的路线规划问题。以下是一个简化的蚁群算法路线规划的代码概述:
```python
# 导入所需库
import numpy as np
import random
# 定义蚂蚁类
class Ant:
def __init__(self, colony, graph):
self.colony = colony
self.graph = graph
self.position = None
self.best_path = []
self pheromone = 0
self.path_cost = float('inf')
# 随机起始位置
def initialize(self):
self.position = random.choice(list(graph.nodes))
# 进行搜索
def search(self):
while not self.colony.stop:
# 选择下一个节点
next_node = self.select_next_node()
# 更新路径和状态
self.update_path(next_node)
return self.best_path
# 选择节点的函数,考虑了pheromone和距离
def select_next_node(self):
neighbors = self.graph.neighbors(self.position)
probabilities = [self.pheromone[node] / (self.pheromone[node] + graph[node].weight) for node in neighbors]
total_prob = sum(probabilities)
probabilities = [prob / total_prob for prob in probabilities]
return random.choices(neighbors, probabilities)
# 更新路径和状态
def update_path(self, next_node):
if next_node not in self.best_path or self.graph.get_weight(self.position, next_node) < self.path_cost:
self.path_cost = self.graph.get_weight(self.position, next_node)
self.best_path.append(next_node)
self.position = next_node
self.colony.update_pheromone(self.position)
# 定义蚁群类
class AntColony:
def __init__(self, graph, ants_count, iterations):
self.graph = graph
self.ants = [Ant(self, graph) for _ in range(ants_count)]
self.iterations = iterations
self.pheromone_matrix = np.ones((graph.nodes_count, graph.nodes_count)) # 初始化pheromone矩阵
self.stop = False
# 更新pheromone值
def update_pheromone(self, node):
# 更新节点间的pheromone
# 实现自适应更新规则
...
# 主循环
def run(self):
for _ in range(self.iterations):
for ant in self.ants:
ant.initialize()
ant.search()
self.update_pheromone(ant.position)
return self.get_best_path()
# 示例使用
if __name__ == "__main__":
graph = create_graph() # 创建图数据结构
ant_colony = AntColony(graph, ants_count=100, iterations=1000)
best_path = ant_colony.run()
print("Best found path:", best_path)
阅读全文
相关推荐
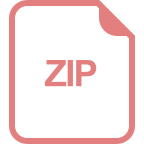
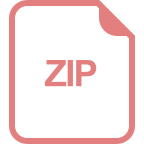
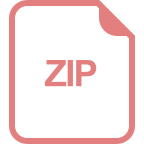
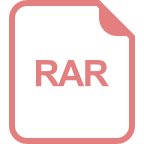
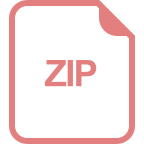
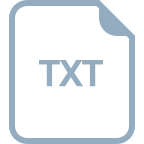
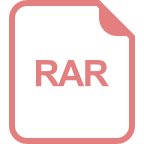
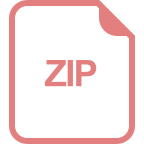
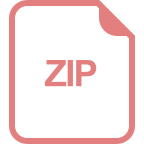
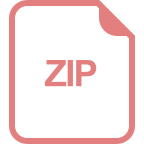
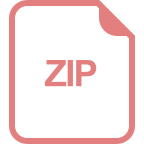
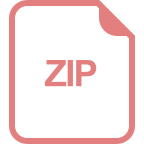
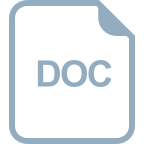
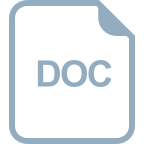
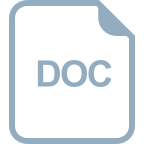
