如何使用python实现一个简单的人脸匹配
时间: 2023-06-14 22:05:19 浏览: 110
要实现一个简单的人脸匹配,需要使用人脸识别技术和机器学习算法。下面是一个基本的人脸匹配的步骤:
1. 收集数据集:收集一些已知的人脸图片,这些图片需要包含不同人的正面照片。可以使用公共的数据集,如LFW数据集。
2. 特征提取:使用人脸识别算法提取每张图片的特征向量。可以使用OpenCV 或 DLib等库进行人脸识别和特征提取。
3. 建立模型:使用机器学习算法,如支持向量机(SVM)或神经网络,训练一个模型,该模型将输入的特征向量映射到特定的人。
4. 预测:使用训练好的模型进行预测。对于一个新的人脸,提取其特征向量并输入到模型中进行预测,得到最相似的人。
下面是一个简单的Python代码实现:
```python
import cv2
import dlib
import numpy as np
import os
# 收集数据集
dataset_path = 'dataset'
face_detector = dlib.get_frontal_face_detector()
face_reco_model = dlib.face_recognition_model_v1('models/dlib_face_recognition_resnet_model_v1.dat')
descriptors = []
images = []
for f in os.listdir(dataset_path):
if f.endswith('.jpg') or f.endswith('.png'):
img_path = os.path.join(dataset_path, f)
img = cv2.imread(img_path)
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
dets = face_detector(img, 1)
for k, d in enumerate(dets):
shape = face_reco_model(img, d)
face_descriptor = np.array(face_reco_model.compute_face_descriptor(img, shape))
descriptors.append(face_descriptor)
images.append((img, shape))
# 建立模型
X = np.array(descriptors)
y = np.array([0] * len(X))
model = cv2.ml.SVM_create()
model.setType(cv2.ml.SVM_C_SVC)
model.setKernel(cv2.ml.SVM_LINEAR)
model.train(X, cv2.ml.ROW_SAMPLE, y)
# 预测
test_img_path = 'test.jpg'
test_img = cv2.imread(test_img_path)
test_img = cv2.cvtColor(test_img, cv2.COLOR_BGR2RGB)
dets = face_detector(test_img, 1)
for k, d in enumerate(dets):
shape = face_reco_model(test_img, d)
face_descriptor = np.array(face_reco_model.compute_face_descriptor(test_img, shape))
test_X = np.array([face_descriptor])
_, result = model.predict(test_X)
print('this person is:', result)
```
在这个例子中,我们收集了一个数据集,使用DLib库进行特征提取,然后使用OpenCV库建立了一个SVM模型。最后,我们使用模型预测一个新的人脸是哪个人。需要注意的是,这只是一个简单的例子,实际应用中可能需要更多的优化和改进。
阅读全文
相关推荐
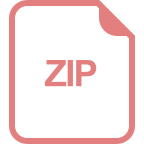
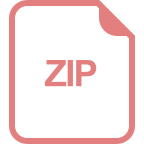
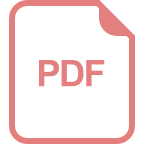















